如何用python编写抢购程序
时间: 2024-06-08 16:09:11 浏览: 202
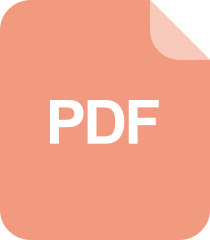
Python实现抢购IPhone手机
编写抢购程序需要先确定购买的商品和购买渠道,然后通过网络请求模拟用户行为进行自动化操作。以下是一个简单的使用 Python 实现的抢购程序的示例:
```python
import requests
import time
# 商品信息
product_id = '123456'
product_url = 'https://www.example.com/product/' + product_id
# 用户信息
user_agent = 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
cookies = {
'cookie1': 'value1',
'cookie2': 'value2'
}
# 抢购函数
def buy():
# 模拟用户打开商品页面
headers = {
'User-Agent': user_agent,
'Referer': product_url
}
response = requests.get(product_url, headers=headers, cookies=cookies)
# 模拟用户提交订单
headers = {
'User-Agent': user_agent,
'Referer': product_url,
'Content-Type': 'application/x-www-form-urlencoded'
}
data = {
'product_id': product_id,
'quantity': '1'
}
response = requests.post('https://www.example.com/order', headers=headers, cookies=cookies, data=data)
# 检查订单状态
if response.status_code == 200 and 'success' in response.text:
print('购买成功')
else:
print('购买失败')
# 循环抢购
while True:
# 获取当前时间
now = time.localtime()
if now.tm_hour == 10 and now.tm_min == 0 and now.tm_sec == 0:
# 在指定时间开始抢购
buy()
break
# 延时1秒钟
time.sleep(1)
```
在这个示例中,我们首先定义了要购买的商品信息和用户信息,然后编写了一个名为 `buy` 的函数来模拟用户进行抢购操作。在主程序中,我们通过循环不断检查当前时间,当到达指定的时间时就开始抢购。当然,这只是一个简单示例,实际的抢购程序需要考虑更多的因素,例如网络延迟、验证码识别等等。
阅读全文
相关推荐
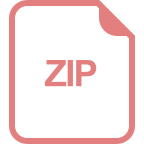
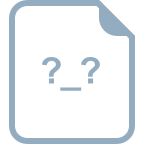

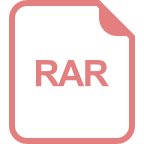
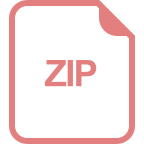






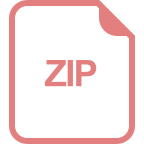
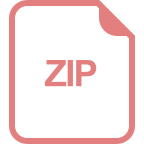
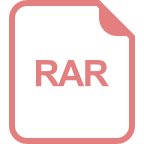


