vue3+vite前端项目,<script setup lang=“ts”>中,iframe嵌套了部分菜单路由,加载过程路由页面中出现白屏闪一下,如何在iframe加载期间,给其设置一个临时的背景颜色或渐变
时间: 2024-10-16 08:15:44 浏览: 10
在 Vue3 使用 Vite 的项目中,如果你遇到了 iframe 内部嵌套菜单路由加载时的白屏闪烁问题,并想设置一个临时背景颜色或渐变直到内容完全加载完成,可以尝试以下步骤:
1. **使用 `IntersectionObserver`**:
使用 JavaScript 的 `Intersection Observer API` 可以监听元素是否进入视口。当 iframe 入口元素可见时,你可以切换其背景样式。
```html
<template>
<div ref="iframeRef">
<iframe :src="iframeSrc" ref="iframeElement"></iframe>
</div>
</template>
<script setup lang="ts">
import { ref, onMounted } from 'vue';
const iframeRef = ref(null);
const iframeSrc = 'your_iframe_src';
onMounted(() => {
const observer = new IntersectionObserver((entries) => {
if (entries[0].isIntersecting) {
// 当iframe可见时,更改背景颜色或渐变
iframeRef.value.style.background = 'linear-gradient(to bottom, #ccc, #fff)';
// 或者直接设置固定颜色
// iframeRef.value.style.background = '#f5f5f5';
observer.unobserve(iframeRef.value); // 停止观察,防止过度操作
}
});
observer.observe(iframeRef.value);
});
</script>
```
2. **使用 Vue 生命周期钩子**:
如果你不想使用第三方库,也可以利用 Vue 的生命周期钩子 `beforeRouteEnter` 来处理这个场景,但这需要你有对路由系统的控制权。
```html
<template>
<router-view :key="route.meta?.name || route.name" :v-if="loading"></router-view>
</template>
<script setup lang="ts">
import { ref, computed, watch } from 'vue';
const loading = ref(true);
// 假设你在组件里有一个对应的路由元信息对象
const routeMeta = computed(() => ({ name: 'your_route_name', backgroundLoadingColor: '#f5f5f5' }));
watch(routeMeta, (newMeta) => {
if (!newMeta) return;
if (newMeta.backgroundLoadingColor) {
loading.value = true;
setTimeout(() => {
document.getElementById('yourIframeId').style.backgroundColor = newMeta.backgroundLoadingColor;
// 或者其他设置背景的方式
loading.value = false;
}, 500); // 设置一定延迟避免瞬间切换导致闪烁
}
}, {
immediate: true,
deep: true,
});
</script>
```
记得替换上述代码中的 `'your_iframe_id'`, `'your_route_name'`, 和 `'your_background_loading_color'` 为实际的 iframe ID、路由名称和你需要的颜色值。
阅读全文
相关推荐
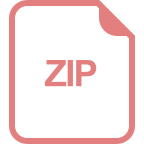
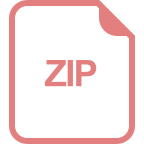
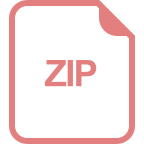















