Python代码人脸校正:根据检测图像中的人脸朝向,将人脸图像校正为正面、上下对称的标准头像。具体实现方法可以采用基于仿射变换的图像校正算法,如Delaunay三角剖分算法。
时间: 2024-05-06 12:22:13 浏览: 16
以下是一种使用Delaunay三角剖分算法实现人脸校正的Python代码:
```python
import cv2
import numpy as np
import dlib
# 初始化dlib的人脸检测器和面部关键点检测器
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor('shape_predictor_68_face_landmarks.dat')
# 定义函数,用于获取人脸关键点坐标
def get_landmarks(image):
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
rects = detector(gray, 1)
if len(rects) == 0:
return None
shape = predictor(gray, rects[0])
landmarks = np.matrix([[p.x, p.y] for p in shape.parts()])
return landmarks
# 定义函数,用于进行人脸校正
def align_face(image):
landmarks = get_landmarks(image)
if landmarks is None:
return None
# 根据左眼、右眼、嘴巴的位置计算人脸朝向
left_eye = landmarks[36:42]
right_eye = landmarks[42:48]
mouth = landmarks[48:68]
# 计算左眼、右眼、嘴巴的中心坐标
left_eye_center = np.mean(left_eye, axis=0).astype('int')
right_eye_center = np.mean(right_eye, axis=0).astype('int')
mouth_center = np.mean(mouth, axis=0).astype('int')
# 计算人脸的朝向向量
eye_vector = right_eye_center - left_eye_center
angle = np.arctan2(eye_vector[1], eye_vector[0]) * 180 / np.pi
# 计算仿射变换矩阵
scale = 1
M = cv2.getRotationMatrix2D(tuple(mouth_center), angle, scale)
# 进行仿射变换
aligned_face = cv2.warpAffine(image, M, (image.shape[1], image.shape[0]))
# 水平翻转校正后的人脸图像,使其上下对称
aligned_face = cv2.flip(aligned_face, 1)
return aligned_face
# 加载测试图像
image = cv2.imread('test.jpg')
# 进行人脸校正
aligned_image = align_face(image)
# 显示校正前后的图像
cv2.imshow('Original Image', image)
cv2.imshow('Aligned Image', aligned_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在代码中,首先使用dlib库中的人脸检测器和面部关键点检测器获取人脸关键点坐标,然后根据左眼、右眼、嘴巴的位置计算人脸的朝向向量,进而计算仿射变换矩阵。最后,使用cv2库中的warpAffine函数进行仿射变换,将人脸校正为正面、上下对称的标准头像。
相关推荐
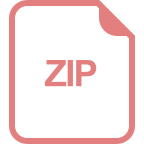
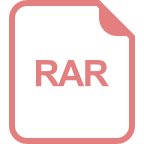
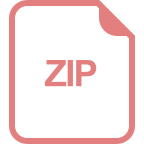













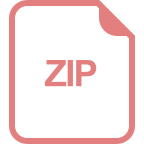
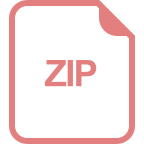