用c语言编程,将两个字符串连接起来但不用strcat
时间: 2023-12-21 10:05:12 浏览: 28
你可以使用指针来实现字符串连接,具体步骤如下:
1. 定义一个新字符串,长度为两个字符串长度之和加一(多加一位用于存放字符串结束标志'\0')。
2. 定义两个指向原字符串和新字符串的指针,分别指向原字符串的首地址和新字符串的首地址。
3. 将原字符串的内容复制到新字符串中。
4. 将原字符串指针和新字符串指针分别移动到字符串末尾。
5. 将第二个原字符串的内容复制到新字符串中。
6. 在新字符串末尾添加结束标志'\0'。
7. 返回新字符串的首地址。
下面是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char* str_connect(char* str1, char* str2)
{
int len1 = strlen(str1);
int len2 = strlen(str2);
char* new_str = (char*)malloc(sizeof(char) * (len1 + len2 + 1));
if (!new_str) {
printf("Error: memory allocation failed!\n");
exit(1);
}
char* p1 = str1;
char* p2 = new_str;
while (*p1) {
*p2++ = *p1++;
}
p1 = str2;
while (*p1) {
*p2++ = *p1++;
}
*p2 = '\0';
return new_str;
}
int main()
{
char str1[] = "Hello, ";
char str2[] = "world!";
char* new_str = str_connect(str1, str2);
printf("%s\n", new_str);
free(new_str);
return 0;
}
```
输出结果为:Hello, world!
相关推荐
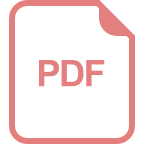
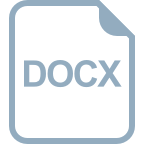
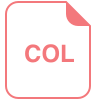
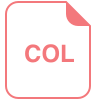
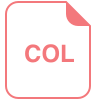
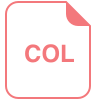
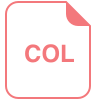









