用PYQT写一个图像处理页面
时间: 2023-12-18 11:05:43 浏览: 109
以下是一个简单的图像处理页面示例,使用Python和PyQt实现。
```python
import sys
import os
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QPushButton, QFileDialog, QVBoxLayout
from PyQt5.QtGui import QPixmap
from PyQt5.QtCore import Qt
import cv2
class ImageProcessor(QWidget):
def __init__(self):
super().__init__()
# 设置窗口大小和标题
self.setWindowTitle("Image Processor")
self.setGeometry(100, 100, 600, 400)
# 创建标签和按钮
self.label = QLabel(self)
self.label.setAlignment(Qt.AlignCenter)
self.button1 = QPushButton("打开图片", self)
self.button2 = QPushButton("灰度化", self)
self.button3 = QPushButton("反转", self)
self.button4 = QPushButton("保存图片", self)
# 创建垂直布局
layout = QVBoxLayout()
layout.addWidget(self.label)
layout.addWidget(self.button1)
layout.addWidget(self.button2)
layout.addWidget(self.button3)
layout.addWidget(self.button4)
# 将布局设置到窗口
self.setLayout(layout)
# 绑定按钮点击事件
self.button1.clicked.connect(self.open_image)
self.button2.clicked.connect(self.grayscale)
self.button3.clicked.connect(self.invert)
self.button4.clicked.connect(self.save_image)
# 初始化变量
self.image_path = ""
self.image = None
def open_image(self):
# 打开文件对话框,选择图片
options = QFileDialog.Options()
options |= QFileDialog.DontUseNativeDialog
file_path, _ = QFileDialog.getOpenFileName(self, "打开图片", "", "All Files (*);;Image Files (*.png *.jpg *.jpeg *.bmp)", options=options)
if file_path:
# 加载图片并显示
self.image_path = file_path
self.image = cv2.imread(self.image_path)
pixmap = QPixmap(self.image_path)
self.label.setPixmap(pixmap)
def grayscale(self):
# 将图片转换为灰度图并显示
if self.image is not None:
gray = cv2.cvtColor(self.image, cv2.COLOR_BGR2GRAY)
self.image = gray
height, width = gray.shape
bytes_per_line = width
q_image = QImage(gray.data, width, height, bytes_per_line, QImage.Format_Grayscale8)
pixmap = QPixmap.fromImage(q_image)
self.label.setPixmap(pixmap)
def invert(self):
# 反转图片并显示
if self.image is not None:
inv = cv2.bitwise_not(self.image)
self.image = inv
height, width, channel = inv.shape
bytes_per_line = 3 * width
q_image = QImage(inv.data, width, height, bytes_per_line, QImage.Format_RGB888)
pixmap = QPixmap.fromImage(q_image)
self.label.setPixmap(pixmap)
def save_image(self):
# 保存图片到文件
if self.image is not None and self.image_path:
file_path, _ = QFileDialog.getSaveFileName(self, "保存图片", os.path.splitext(self.image_path)[0] + "_processed.jpg", "JPEG (*.jpg *.jpeg)")
if file_path:
cv2.imwrite(file_path, self.image)
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = ImageProcessor()
ex.show()
sys.exit(app.exec_())
```
这个页面包括以下功能:
- 打开图片按钮:打开文件对话框,选择一张图片并显示在标签中。
- 灰度化按钮:将图片转换为灰度图并显示在标签中。
- 反转按钮:将图片颜色反转并显示在标签中。
- 保存图片按钮:将处理后的图片保存到文件。
这是一个简单的示例,您可以根据自己的需求对页面进行更改和扩展。
阅读全文
相关推荐
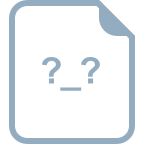
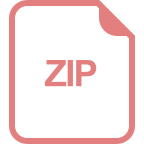
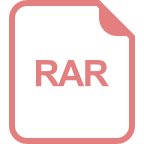
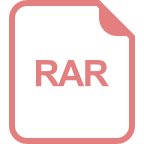
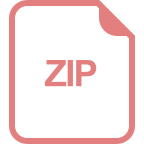
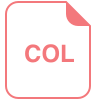









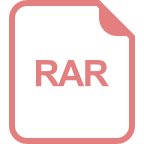
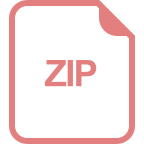
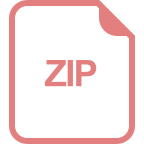