写一个字符数组排序的算法
时间: 2024-05-10 19:15:49 浏览: 6
一个简单的字符数组排序算法是冒泡排序。它的基本思想是重复遍历数组,比较相邻的元素并交换它们,直到没有需要交换的元素为止。
下面是一个使用冒泡排序算法对字符数组进行排序的示例代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
void bubbleSort(char arr[], int n) {
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
char temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
int main() {
char arr[] = "hello world";
int n = strlen(arr);
bubbleSort(arr, n);
cout << "Sorted array: ";
for (int i = 0; i < n; i++) {
cout << arr[i];
}
cout << endl;
return 0;
}
```
该算法的时间复杂度为 O(n^2),因此在大型数组上的性能可能会受到限制。
相关推荐
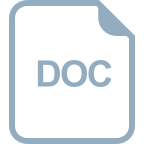
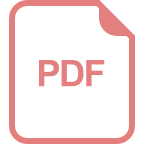














