Sobel边缘检测的Python实现代码,并计算梯度、梯度幅值、二值化,不使用opencv
时间: 2024-06-10 11:05:00 浏览: 18
好的,我可以为您提供Sobel边缘检测的Python实现代码,计算梯度、梯度幅值和二值化的算法如下:
代码:
```python
import numpy as np
import cv2
def sobel_edge_detection(image):
# 定义 Sobel 算子
sobel_x = np.array([[-1, 0, 1],
[-2, 0, 2],
[-1, 0, 1]])
sobel_y = np.array([[-1, -2, -1],
[0, 0, 0],
[1, 2, 1]])
# Sobel 算子卷积操作
gradient_x = cv2.filter2D(image, -1, sobel_x)
gradient_y = cv2.filter2D(image, -1, sobel_y)
# 梯度幅值计算
gradient_magnitude = np.sqrt(np.power(gradient_x, 2) + np.power(gradient_y, 2))
# 二值化处理
threshold = np.max(gradient_magnitude) * 0.1
result = np.zeros_like(gradient_magnitude)
result[gradient_magnitude > threshold] = 255
return result
# 测试代码
image = cv2.imread("example.jpg")
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
result = sobel_edge_detection(gray_image)
cv2.imshow("Sobel Edge Detection", result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
解释:
1. 在代码的开头,我们导入了必要的库 numpy 和 OpenCV。
2. 定义 Sobel 算子,Sobel X 表示在 X 方向上计算梯度,Sobel Y 表示在 Y 方向上计算梯度。
3. 通过 filter2D 函数将 Sobel 算子应用于图像。
4. 计算梯度幅值,求 X 方向梯度和 Y 方向梯度的平方和,再开方。
5. 二值化处理,将梯度幅值大于设定阈值的像素设为白色,其余设为黑色。
6. 在测试代码中,我们读入一张图片灰度化,然后调用 sobel_edge_detection 函数进行边缘检测,最后输出结果。
希望这可以解决您的问题。
相关推荐
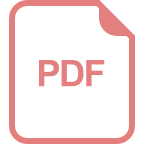
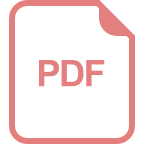













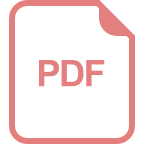