用python编写一个程序 输入年和月,生成输入年月的日历表。
时间: 2024-03-07 16:47:21 浏览: 80
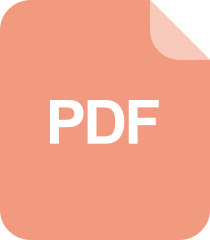
python实现输入任意一个大写字母生成金字塔的示例

可以使用Python中的calendar模块来生成日历表。以下是一个简单的程序,可以根据用户输入的年份和月份生成对应的日历表。
```python
import calendar
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
# 使用calendar模块的monthrange函数获取指定月份的天数
days = calendar.monthrange(year, month)[1]
# 使用calendar模块的month函数生成指定月份的日历表
cal = calendar.month(year, month)
print("以下是%d年%d月的日历表:" % (year, month))
print(cal)
```
运行程序后,用户需要输入要查询的年份和月份,程序会根据用户输入的年份和月份生成对应月份的日历表。例如,输入年份为2021,月份为5,程序会输出以下结果:
```
请输入年份:2021
请输入月份:5
以下是2021年5月的日历表:
May 2021
Mo Tu We Th Fr Sa Su
1 2
3 4 5 6 7 8 9
10 11 12 13 14 15 16
17 18 19 20 21 22 23
24 25 26 27 28 29 30
31
```
阅读全文
相关推荐
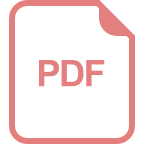
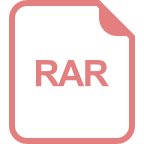















