java GUI和MySQL编程程序实现模拟某学籍系统的注册和登录功能:注册时需要检查其输入的12位学号是否全是数字(前4位是2005-2022范围内的年份)且不和已注册的学号重复,密码是6-12位的字符,【选做功能:可以采用随机生成的图片作为为验证码】,注册成功则显示成功提示,否则出现相应的错误提醒;登录时根据具体登录情况显示成功或相应的错误信息。要求该程序采用GUI界面,且将学生的学号及登录密码保存在一个数据表中,实验内容应展示数据库及数据表的设计等相关信息的代码
时间: 2024-03-02 21:51:17 浏览: 69
好的,我可以为您提供一些思路和代码。首先,需要设计一个MySQL数据表来保存学生的学号和密码信息。可以使用以下SQL语句创建一个名为 `students` 的数据表:
```sql
CREATE TABLE students (
id INT(11) NOT NULL AUTO_INCREMENT,
student_id VARCHAR(12) NOT NULL,
password VARCHAR(12) NOT NULL,
PRIMARY KEY (id)
);
```
接下来,需要编写Java GUI程序来实现注册和登录功能。下面是一个基本的示例程序:
```java
import java.awt.EventQueue;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class StudentSystemGUI {
private JFrame frame;
private JTextField idField;
private JTextField passwordField;
private Connection conn;
private PreparedStatement stmt;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
StudentSystemGUI window = new StudentSystemGUI();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public StudentSystemGUI() {
initialize();
connectDB();
}
private void initialize() {
frame = new JFrame("学籍系统");
frame.setBounds(100, 100, 450, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().setLayout(null);
JPanel panel = new JPanel();
panel.setBounds(10, 10, 414, 243);
frame.getContentPane().add(panel);
panel.setLayout(null);
JLabel idLabel = new JLabel("学号");
idLabel.setFont(new Font("宋体", Font.PLAIN, 16));
idLabel.setBounds(50, 50, 50, 30);
panel.add(idLabel);
idField = new JTextField();
idField.setBounds(110, 50, 200, 30);
panel.add(idField);
idField.setColumns(10);
JLabel passwordLabel = new JLabel("密码");
passwordLabel.setFont(new Font("宋体", Font.PLAIN, 16));
passwordLabel.setBounds(50, 100, 50, 30);
panel.add(passwordLabel);
passwordField = new JTextField();
passwordField.setColumns(10);
passwordField.setBounds(110, 100, 200, 30);
panel.add(passwordField);
JButton registerButton = new JButton("注册");
registerButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
register();
}
});
registerButton.setBounds(50, 170, 100, 30);
panel.add(registerButton);
JButton loginButton = new JButton("登录");
loginButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
login();
}
});
loginButton.setBounds(210, 170, 100, 30);
panel.add(loginButton);
}
private void connectDB() {
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/student_system", "root", "password");
stmt = conn.prepareStatement("SELECT * FROM students WHERE student_id = ?");
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
}
private void register() {
String id = idField.getText();
String password = passwordField.getText();
if (!id.matches("^20[0-9]{2}[0-9]{8}$")) {
JOptionPane.showMessageDialog(frame, "学号必须是12位数字,前4位是2005-2022范围内的年份。");
return;
}
try {
stmt.setString(1, id);
ResultSet rs = stmt.executeQuery();
if (rs.next()) {
JOptionPane.showMessageDialog(frame, "该学号已经被注册。");
return;
}
} catch (SQLException e) {
e.printStackTrace();
}
if (password.length() < 6 || password.length() > 12) {
JOptionPane.showMessageDialog(frame, "密码必须是6-12位的字符。");
return;
}
try {
PreparedStatement insertStmt = conn.prepareStatement("INSERT INTO students (student_id, password) VALUES (?, ?)");
insertStmt.setString(1, id);
insertStmt.setString(2, password);
insertStmt.executeUpdate();
JOptionPane.showMessageDialog(frame, "注册成功。");
} catch (SQLException e) {
e.printStackTrace();
JOptionPane.showMessageDialog(frame, "注册失败,请重试。");
}
}
private void login() {
String id = idField.getText();
String password = passwordField.getText();
try {
stmt.setString(1, id);
ResultSet rs = stmt.executeQuery();
if (rs.next()) {
if (password.equals(rs.getString("password"))) {
JOptionPane.showMessageDialog(frame, "登录成功。");
} else {
JOptionPane.showMessageDialog(frame, "密码错误,请重试。");
}
} else {
JOptionPane.showMessageDialog(frame, "该学号未注册,请先注册。");
}
} catch (SQLException e) {
e.printStackTrace();
JOptionPane.showMessageDialog(frame, "登录失败,请重试。");
}
}
}
```
在这个程序中,我们首先创建了一个名为 `students` 的数据表来保存学生的学号和密码信息。然后,我们使用Java Swing框架创建了一个GUI界面,并添加了用于输入学号和密码的文本框,以及注册和登录按钮。在注册和登录功能实现中,我们使用了MySQL的JDBC驱动程序来连接数据库,并执行相应的SQL语句来检查学号和密码是否符合要求,并保存或检索学生信息。最后,我们使用JOptionPane类来显示相应的提示信息。
当然,这只是一个简单的示例程序,您可以根据具体需求和业务逻辑进行相应的修改和优化。
阅读全文
相关推荐
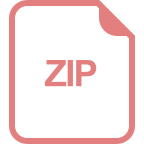
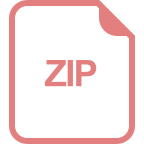
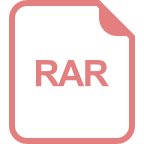
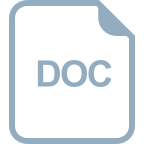
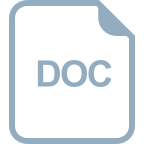
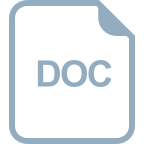
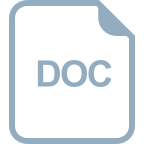
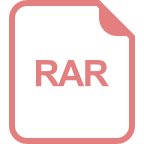
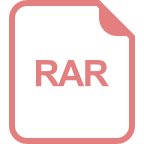
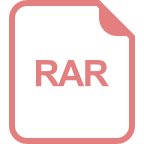
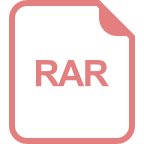
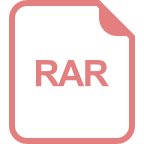
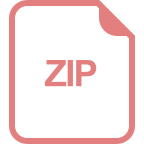
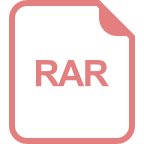
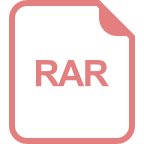
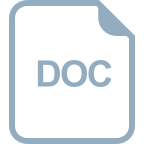