高德地图api获取两个 坐标之间的直线距离
时间: 2024-09-15 14:03:55 浏览: 51
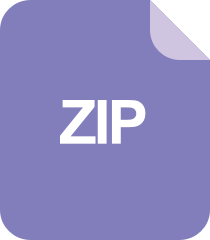
高德地图路径规划api,批量获取坐标点间的距离,驾车时间等
在高德地图 API 中,获取两点之间的直线距离可以通过 `AMap.Geolocation` 和 `AMap.Polyline` 组件结合来实现。以下是一个简单的步骤:
1. **引入依赖库**:首先确保已导入高德地图相关的 JavaScript 库和模块。
```javascript
import AMap from 'amap-js-sdk';
```
2. **初始化地图实例**:在需要的地方创建地图实例。
```javascript
const map = new AMap.Map('container', {
zoom: 10, // 地图初始缩放级别
center: [116.39, 39.9], // 北京的中心坐标
});
```
3. **获取地理编码服务**:用于将地址转换为经纬度。
```javascript
const geocoder = new AMap.Geocoder();
```
4. **查询起点和终点的经纬度**:根据实际需求,用户输入或从数据库获取地址,调用 `geocode()` 方法。
```javascript
function getDistance(startAddress, endAddress) {
return new Promise((resolve, reject) => {
geocoder.getAddress(startAddress, (status, result) => {
if (status === 'complete' && result.info === 'OK') {
const startPoint = result.formatted_addresses;
geocoder.getAddress(endAddress, (status, result) => {
if (status === 'complete' && result.info === 'OK') {
const endPoint = result.formatted_addresses;
// 获取经纬度数组
const startCoord = result.poi ? result.poi.location : result.addressComponent.lnglat;
const endCoord = result.poi ? result.poi.location : result.addressComponent.lnglat;
// 计算距离
calculateLineDistance(startCoord, endCoord).then(distance => {
resolve(distance);
});
} else {
reject(result.message);
}
});
} else {
reject(result.message);
}
});
});
}
// 自定义方法计算两点间的直线距离
function calculateLineDistance(startCoord, endCoord) {
const startLat = startCoord.lat,
startLng = startCoord.lng,
endLat = endCoord.lat,
endLng = endCoord.lng;
const distanceService = new AMap.DistanceMatrix();
distanceService.calculate([
{ location: startLat + ',' + startLng },
{ location: endLat + ',' + endLng }
]).then(response => {
const result = response.result;
return result.distance.toFixed(2); // 返回千米为单位的距离
}).catch(err => console.error(err));
}
```
5. **调用距离计算**:当你需要知道两点之间距离时,传入地址并处理返回结果。
```javascript
getDistance('起点地址', '终点地址')
.then(distance => {
console.log(`两点之间的直线距离是 ${distance} 千米`);
})
.catch(error => {
console.error('获取距离失败:', error);
});
```
注意:此代码示例假设你已经在 HTML 文件中添加了名为 "container" 的 div 元素供地图渲染,并且已经设置了 API 密钥。此外,实际项目中可能还需要考虑网络请求的错误处理和性能优化。
阅读全文
相关推荐
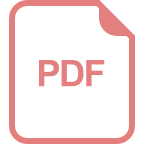
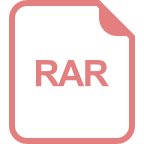

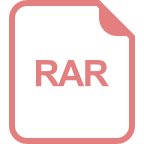
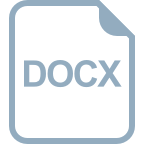
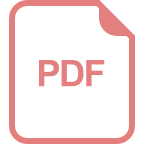
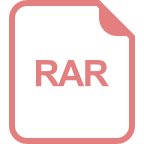
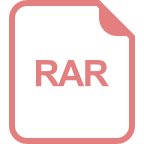
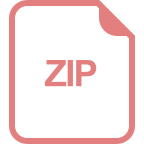
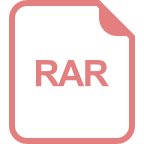
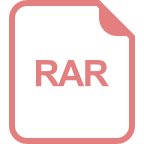
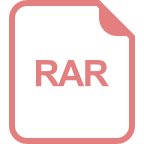
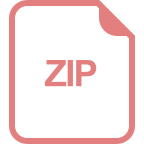
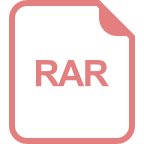

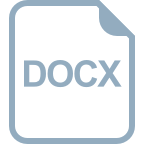