思考: 有一个充分大的池子,两个人分别向池中扔球。甲扔红球,乙扔兰球,一次扔一个。开始时池中有红球、兰球各一个,要求池中球满足条件: 1<=红球数/兰球数<=2 试用P、V描述两个过程。c语言
时间: 2024-09-29 11:15:40 浏览: 69
为了实现这个场景,我们可以使用互斥量(mutex)和信号量(semaphore)来协调两个玩家的操作,以保持红球和蓝球的比例在1到2之间。这里假设我们使用的是 C 语言中的 Pthread 模块。
首先,定义两个信号量:
1. `red_semaphore`:表示红球的数量,初始化为1(因为一开始有1个红球)。
2. `blue_semaphore`:表示蓝球的数量,初始化为1(同样是因为初始有1个蓝球)。
接着,定义互斥锁(mutex)`pool_mutex`,用于保护对信号量的访问。
现在,我们可以定义两个过程,分别是甲(throw_red)和乙(throw_blue),它们分别代表扔红球和蓝球的过程:
```c
#include <pthread.h>
#include <semaphore.h>
#define MAX_RATIO 2
#define SEMAPHORES (MAX_RATIO + 1) // 最大红球比蓝球数
sem_t red_semaphore;
sem_t blue_semaphore;
pthread_mutex_t pool_mutex;
void *throw_red(void *arg) {
int ball = 1; // 红球计数
while (true) {
pthread_mutex_lock(&pool_mutex);
if (ball <= SEMAPHORES && ball > blue_semaphore.val) { // 当红球少于蓝球,且红球不多于最大比例
sem_post(&red_semaphore); // 允许放回红球
sem_wait(&blue_semaphore); // 获取蓝球权限
sem_post(&blue_semaphore); // 放回蓝球
ball++;
} else {
sem_wait(&red_semaphore); // 等待获取红球权限
}
pthread_mutex_unlock(&pool_mutex);
// ... 实际投掷操作 ...
// 如果不再需要红球,结束循环
if (/*满足停止条件*/) {
break;
}
}
return NULL;
}
void *throw_blue(void *arg) {
int ball = 1; // 蓝球计数
while (true) {
pthread_mutex_lock(&pool_mutex);
if (ball > red_semaphore.val) { // 当蓝球超过红球
sem_post(&blue_semaphore); // 允许放回蓝球
sem_wait(&red_semaphore); // 获取红球权限
sem_post(&red_semaphore); // 放回红球
ball--;
} else {
sem_wait(&blue_semaphore); // 等待获取蓝球权限
}
pthread_mutex_unlock(&pool_mutex);
// ... 实际投掷操作 ...
// 如果不再需要蓝球,结束循环
if (/*满足停止条件*/) {
break;
}
}
return NULL;
}
int main() {
// 初始化信号量和互斥锁
sem_init(&red_semaphore, 0, 1);
sem_init(&blue_semaphore, 0, 1);
pthread_mutex_init(&pool_mutex, NULL);
// 创建线程并启动
pthread_create(&thread_red, NULL, throw_red, NULL);
pthread_create(&thread_blue, NULL, throw_blue, NULL);
// 等待所有线程完成
pthread_join(thread_red, NULL);
pthread_join(thread_blue, NULL);
// 清理资源
sem_destroy(&red_semaphore);
sem_destroy(&blue_semaphore);
pthread_mutex_destroy(&pool_mutex);
return 0;
}
```
阅读全文
相关推荐
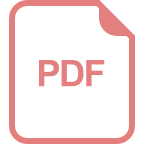
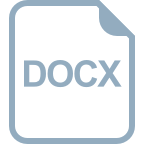
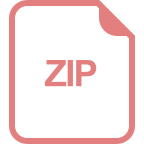
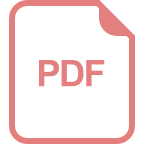
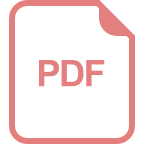
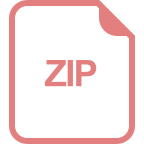
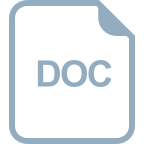
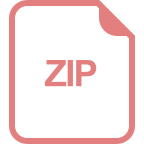
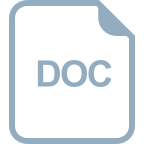
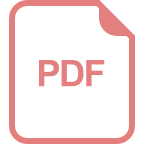
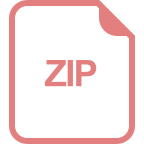
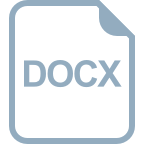
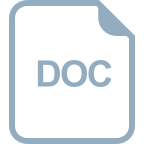
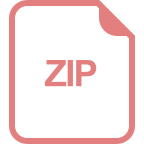
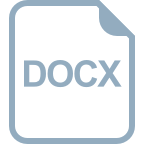
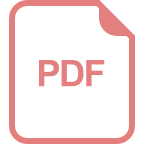