python最短路径问题动态规划
时间: 2024-12-06 11:14:39 浏览: 10
动态规划是一种通过将复杂问题分解为更简单的子问题来解决问题的方法。在最短路径问题中,动态规划可以用来找到从起点到终点的最短路径。Python中可以使用动态规划来解决最短路径问题,下面是一个简单的示例:
假设我们有一个图,图的每个节点都有一个权重,我们希望找到从起点到终点的最短路径。我们可以使用动态规划来解决这个问题。
```python
def shortest_path(graph, start, end):
# 创建一个字典来存储从起点到每个节点的最短路径
distances = {vertex: float('inf') for vertex in graph}
distances[start] = 0
# 创建一个集合来存储已经计算过的节点
visited = set()
while True:
# 找到当前未访问节点中距离起点最近的节点
current_vertex = None
for vertex in graph:
if vertex not in visited and (current_vertex is None or distances[vertex] < distances[current_vertex]):
current_vertex = vertex
if current_vertex is None:
break
# 标记当前节点为已访问
visited.add(current_vertex)
# 更新当前节点的邻居节点的距离
for neighbor, weight in graph[current_vertex].items():
distance = distances[current_vertex] + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
return distances[end]
# 示例图
graph = {
'A': {'B': 1, 'C': 4},
'B': {'A': 1, 'C': 2, 'D': 5},
'C': {'A': 4, 'B': 2, 'D': 1},
'D': {'B': 5, 'C': 1}
}
start = 'A'
end = 'D'
print(f"从{start}到{end}的最短路径长度为: {shortest_path(graph, start, end)}")
```
在这个示例中,我们定义了一个`shortest_path`函数,它接受一个图、起点和终点作为参数。函数使用动态规划的方法来计算从起点到终点的最短路径。
阅读全文
相关推荐
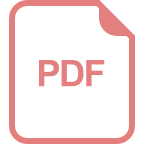
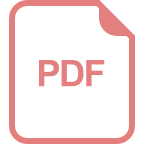
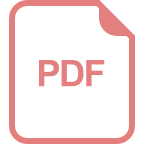
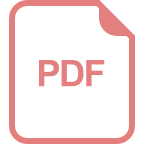

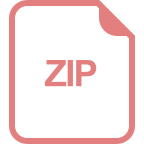
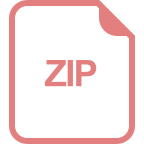
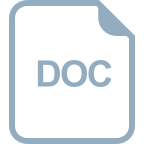










