2.已知有一个包含一些同学成绩的字典,计算成绩的最高分,最低分、平均分, 并查找最高分同学,最后打印最高分,最低分,平均分,最高分同学。
时间: 2024-10-14 22:14:05 浏览: 146
为了完成这个任务,我们可以按照以下步骤设计一个Python程序:
首先,假设我们有一个表示学生和他们成绩的字典结构,例如每个键是学生的姓名,值是一个列表,包含了该学生的所有成绩。这里是一个示例字典:
```python
student_scores = {
"张三": [85, 90, 92],
"李四": [78, 82, 86],
"王五": [98, 95, 97]
}
```
1. 计算最高分和最低分:
- 初始化两个变量`max_score`和`min_score`,分别存储当前找到的最大分数和最小分数。遍历每个学生的成绩列表,更新这两个变量。
```python
max_score = min_score = student_scores.values()[0][0] # 初始值为第一个学生的第一个成绩
for scores in student_scores.values():
if max(scores) > max_score:
max_score = max(scores)
if min(scores) < min_score:
min_score = min(scores)
```
2. 计算平均分:
- 初始化`sum_scores`用于累加总分数,然后除以学生数得到平均分。
```python
sum_scores = sum(score for scores in student_scores.values() for score in scores)
avg_score = sum_scores / len(student_scores)
```
3. 查找最高分同学:
- 使用`max_score`作为键,在字典中查找对应的姓名。
```python
highest_student = next((name for name, scores in student_scores.items() if max(scores) == max_score), None)
if not highest_student:
highest_student = "无"
```
4. 打印结果:
```python
print(f"最高分:{max_score},由{highest_student}获得")
print(f"最低分:{min_score}")
print(f"平均分:{avg_score}")
```
完整代码如下:
```python
student_scores = {
"张三": [85, 90, 92],
"李四": [78, 82, 86],
"王五": [98, 95, 97]
}
max_score = min_score = student_scores.values()[0][0]
highest_student = None
for scores in student_scores.values():
if max(scores) > max_score:
max_score = max(scores)
highest_student = student_scores.keys()[scores.index(max(scores))]
if min(scores) < min_score:
min_score = min(scores)
sum_scores = sum(score for scores in student_scores.values() for score in scores)
avg_score = sum_scores / len(student_scores)
print(f"最高分:{max_score},由{highest_student}获得")
print(f"最低分:{min_score}")
print(f"平均分:{avg_score}")
```
阅读全文
相关推荐
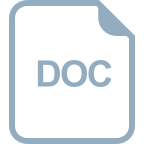
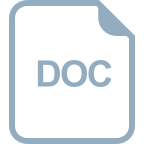
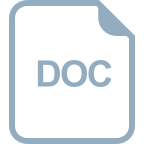













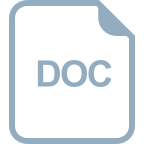
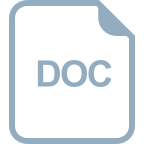
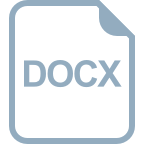