写一个java程序,将json文件转为srt文件
时间: 2024-12-13 12:26:09 浏览: 8
在Java中,你可以使用诸如Jackson库(处理JSON)、Guava库(提供方便的IO操作)以及SimpleDateFormat等内置类来完成JSON到SRT的转换。这里给出一个简化版的示例代码,假设你的JSON文件是简单的键值对,包含“start_time”和“text”。
```java
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.FileWriter;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.List;
public class JsonToSrtConverter {
public static void main(String[] args) {
String jsonFilePath = "input.json";
String srtFilePath = "output.srt";
// 读取JSON文件
ObjectMapper mapper = new ObjectMapper();
List<Subtitle> subtitles = mapper.readValue(new File(jsonFilePath), new ArrayList<>());
// 创建SRT文件并写入
try (BufferedReader reader = new BufferedReader(new FileReader(jsonFilePath));
FileWriter writer = new FileWriter(srtFilePath)) {
for (Subtitle subtitle : subtitles) {
SimpleDateFormat sdf = new SimpleDateFormat("HH:mm:ss,SSS");
String timeStr = sdf.format(subtitle.getTime());
writer.write(timeStr + "\n--> " + subtitle.getText() + "\n\n");
}
} catch (Exception e) {
e.printStackTrace();
}
}
static class Subtitle {
private long time; // 时间戳
private String text;
// getters and setters...
public long getTime() {
return time;
}
public void setTime(long time) {
this.time = time;
}
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
}
}
```
在这个例子中,你需要先定义一个Subtitle类,它应该包含时间和文本字段,以便映射JSON中的数据。然后,通过Jackson库将JSON文件的内容解压到Subtitle对象列表中,再遍历这个列表,按照SRT格式输出。
注意这只是一个基础的示例,实际项目中可能会遇到更复杂的JSON结构,可能需要解析嵌套对象或者数组,那就要调整代码以适应JSON的实际情况。
阅读全文
相关推荐
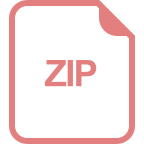
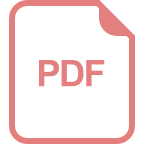
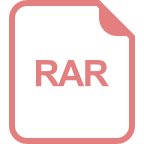
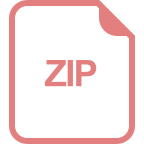
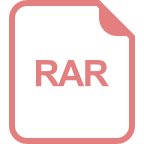
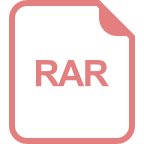
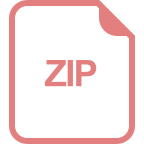
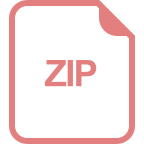
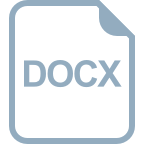
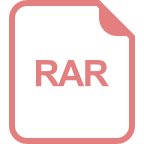
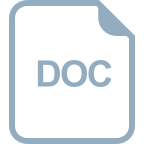
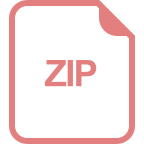
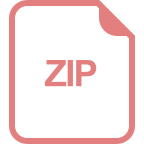
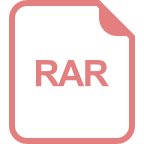
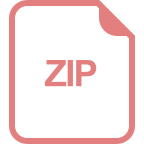
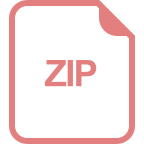
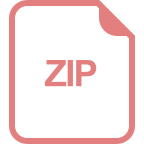