在使用Java和Apache POI解析.docx文档时,如何准确获取文档内图片的具体位置?
时间: 2024-11-01 12:19:26 浏览: 67
在处理.docx文件时,图片的位置信息被嵌入在`document.xml`中,特别是`w:drawing`元素内,它包含了图片的布局和位置属性。为了准确获取图片位置,开发者需要解析这些XML结构并理解.docx文件的内部组成。具体步骤如下:(步骤、代码、mermaid流程图、扩展内容,此处略)
参考资源链接:[Java解析.docx获取图片位置的Apache POI实现](https://wenku.csdn.net/doc/1ypiswsudb?spm=1055.2569.3001.10343)
通过以上方法,可以在Java中使用Apache POI库解析.docx文件并准确地获取到文档内每张图片的位置信息。这个过程需要对Apache POI的XWPF组件有深入的理解,以及对.docx文件结构有一定的认识。如果你希望进一步掌握Apache POI解析.docx文件的高级技术,我强烈推荐你查阅《Java解析.docx获取图片位置的Apache POI实现》这份资料。它提供了详细的理论基础和实战指导,将帮助你更全面地理解文档解析技术,无论是对于当前问题的解决,还是对文档处理领域更深入的学习,这都是一个不可多得的资源。
参考资源链接:[Java解析.docx获取图片位置的Apache POI实现](https://wenku.csdn.net/doc/1ypiswsudb?spm=1055.2569.3001.10343)
相关问题
如何在Java中使用Apache POI解析.docx文档,以准确获取文档内图片的具体位置?
在处理.docx文件时,由于其内部结构为ZIP压缩包格式,直接获取图片位置有一定的难度。利用Apache POI,我们可以访问并解析`document.xml`,找到包含图片布局信息的`w:drawing`元素,进而确定图片在文档中的位置。具体步骤如下:
参考资源链接:[Java解析.docx获取图片位置的Apache POI实现](https://wenku.csdn.net/doc/1ypiswsudb?spm=1055.2569.3001.10343)
首先,使用Apache POI中的`XWPFDocument`类加载.docx文件,然后遍历文档中的所有段落(`XWPFParagraph`)和运行(`XWPFRun`)。在运行中查找包含`w:drawing`元素的实例,这些元素中包含了关于图片的布局信息。
在`w:drawing`元素内部,通常会有一个`wp:inline`元素,它包含了关于图片位置的详细信息。具体来说,`wp:extent`元素表明了图片的尺寸,而`wp:positionH`和`wp:positionV`元素则提供了图片相对于文档左边距和上边距的位置信息。这些位置信息通常以EMUs(英语:English Metric Units)为单位,需要转换成像素或其他单位以便在屏幕上展示。
同时,要获取图片本身的资源文件,可以通过`wp:inline`元素中的`a:blipFill`元素找到图片的标识符,然后在文档的`_rels`文件夹和`media`文件夹中定位到具体的图片文件。
以下是实现上述功能的代码示例:
```java
import org.apache.poi.xwpf.usermodel.*;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.CTPicture;
public class ImagePositionExtractor {
public void extractImagePositions(XWPFDocument doc, String outputFilePath) {
int count = 0;
for (XWPFParagraph p : doc.getParagraphs()) {
for (XWPFRun r : p.getRuns()) {
if (r.getText(0) != null && !r.getText(0).isEmpty()) {
continue;
}
for (XWPFDrawing drawing : r.getDrawings()) {
for (XDPictureData picData : drawing.getRelations()) {
CTPicture pic = drawing.getCTPicture();
// 从pic中获取位置信息,转换成像素,并保存在outputFilePath指向的文件中
}
}
}
}
}
}
```
在上述代码中,我们并没有直接展示如何解析和转换位置信息,因为这需要对Apache POI中的相关类和方法有更深入的了解。建议读者查阅《Java解析.docx获取图片位置的Apache POI实现》这一资料来获得更全面的指导和理解。
总结来说,通过上述方法,我们可以有效地在Java中解析.docx文档,获取并利用图片的位置信息,从而实现更复杂的文档处理任务。
参考资源链接:[Java解析.docx获取图片位置的Apache POI实现](https://wenku.csdn.net/doc/1ypiswsudb?spm=1055.2569.3001.10343)
如何用Java编程语言解析.docx文件中的公式内容?
在Java中解析.docx文件中的公式内容通常涉及到操作Office Open XML (OOXML),这是Microsoft Office 2007及以上版本使用的文件格式标准。要实现这个功能,你需要借助第三方库如Apache POI的XWPF或Aspose.Words等,它们提供了读取和处理Word文档的功能。
以下是一个简单的步骤概述:
1. **添加依赖**:首先,你需要在项目中引入相关的库,如Apache POI。如果你使用Maven,可以在pom.xml文件中添加如下依赖:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.0.0</version>
</dependency>
```
2. **加载文档**:使用`XWPFDocument`类加载.docx文件:
```java
XWPFDocument document = new XWPFDocument(new FileInputStream("path_to_your_docx_file"));
```
3. **查找公式**:公式在.docx中通常是通过`CTMath`元素表示的。你可以遍历文档中的`Body`元素,找到包含数学公式的地方:
```java
for (XWPFParagraph paragraph : document.getParagraphs()) {
for (XWPFRun run : paragraph.getRuns()) {
if (run instanceof CTMath) {
// 这里有公式
}
}
}
```
4. **解析公式**:使用`CTMath`提供的信息提取公式内容,这可能需要一些解析工作,因为公式可能嵌套或包含复杂的表达式。你可能需要用到`CTMmlMath`或`CTFormulaArray`等子元素。
```java
CTMmlMath mmlMath = (CTMmlMath) ((CTInline)(run.getElement())).getChildren().get(0);
String formulaContent = mmlMath.getText();
```
5. **处理公式**:最后,你可以对获取到的公式内容做进一步处理,比如转换成LaTeX、Markdown或其他格式。
记得处理完文件后关闭文档:
```java
document.close();
```
阅读全文
相关推荐
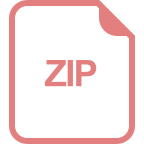
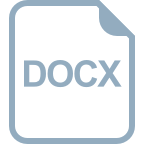
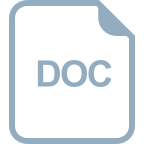
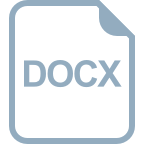
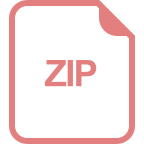
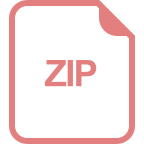
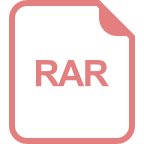
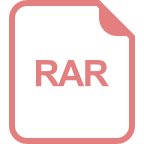
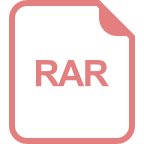
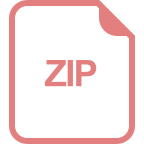
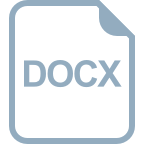
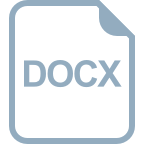
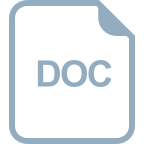


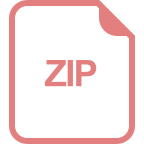