python去除图片背景,使用Python / PIL从图像中删除背景色
时间: 2024-03-05 16:49:18 浏览: 305
要使用Python / PIL从图像中删除背景色,可以按照以下步骤进行:
1. 导入必要的库和模块:
```python
from PIL import Image
import numpy as np
```
2. 打开图像并将其转换为数组:
```python
image = Image.open("image.png")
image_array = np.array(image)
```
3. 将图像数组转换为灰度图像:
```python
gray_array = np.mean(image_array, axis=2).astype(np.uint8)
```
4. 通过阈值化将图像转换为黑白二值图像:
```python
threshold = 150
binary_array = np.where(gray_array > threshold, 255, 0).astype(np.uint8)
```
5. 创建一个掩码图像,将白色像素设置为不透明,将黑色像素设置为透明:
```python
mask_array = np.where(binary_array == 255, 255, 0).astype(np.uint8)
```
6. 创建一个新的RGBA图像并将其掩码设置为新的掩码图像:
```python
new_image_array = np.dstack((image_array, np.full(image_array.shape[:2], 255, dtype=np.uint8)))
new_image_array[:, :, 3] = mask_array
```
7. 将新图像数组转换为图像并保存:
```python
new_image = Image.fromarray(new_image_array, mode='RGBA')
new_image.save("new_image.png")
```
通过以上步骤,你就可以使用Python / PIL从图像中删除背景色了。
阅读全文
相关推荐
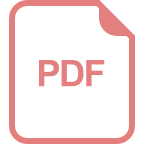
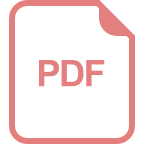
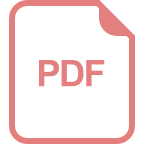
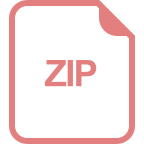
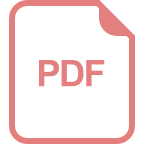
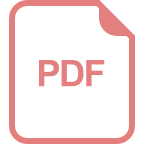
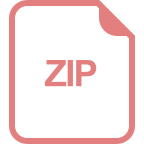
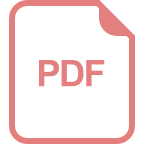
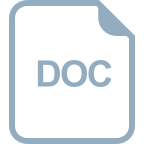



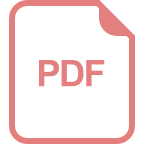
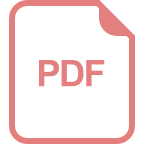
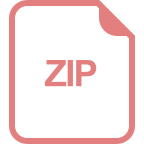
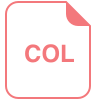

