vector可以储存字符串吗
时间: 2024-10-23 20:09:07 浏览: 26
当然可以。`std::vector` 是 C++ 标准库中的动态数组容器,它可以存储任何类型的对象,包括基本类型、结构体、类实例,甚至是字符串。当你想要用 `vector` 存储字符串时,你可以选择直接存储 `std::string` 类型的元素。
例如:
```cpp
#include <iostream>
#include <vector>
#include <string>
int main() {
std::vector<std::string> myStrings; // 创建一个存放字符串的 vector
myStrings.push_back("Hello"); // 添加一个字符串元素
myStrings.push_back("World");
for (const auto& str : myStrings) {
std::cout << str << " ";
}
return 0;
}
```
在这个例子中,`myStrings` 可以很好地管理字符串序列,并提供添加、删除以及访问字符串的能力。
相关问题
在一个存放字符串的vector容器中,有没有函数可以快速找出里面最长的字符串对应的下标
是的,可以使用`std::max_element`结合`std::string::size()`函数来找到vector中最长字符串对应的下标。
示例代码如下:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
#include <string>
int main() {
std::vector<std::string> vec{"hello", "world", "this", "is", "a", "test"};
auto it = std::max_element(vec.begin(), vec.end(),
[](const std::string& a, const std::string& b) {
return a.size() < b.size();
});
std::cout << "The longest string is " << *it << ", and its index is " << std::distance(vec.begin(), it) << std::endl;
return 0;
}
```
输出:
```
The longest string is hello, and its index is 0
```
、编写主函数,用vector <string>向量存放网页,每一行作为一个元素,用map<string,string>容器存放转换字符串,字符串查找替换均采用string的成员函数实现
### 回答1:
好的,这是一个关于编程的问题。可以回答。以下是代码示例:
#include <iostream>
#include <vector>
#include <string>
#include <map>
using namespace std;
int main()
{
vector<string> webpages;
webpages.push_back("www.google.com");
webpages.push_back("www.facebook.com");
webpages.push_back("www.twitter.com");
map<string, string> convert;
convert["google"] = "谷歌";
convert["facebook"] = "脸书";
convert["twitter"] = "推特";
for (int i = ; i < webpages.size(); i++)
{
string webpage = webpages[i];
for (auto it = convert.begin(); it != convert.end(); it++)
{
string key = it->first;
string value = it->second;
size_t pos = webpage.find(key);
if (pos != string::npos)
{
webpage.replace(pos, key.length(), value);
}
}
cout << webpage << endl;
}
return ;
}
### 回答2:
下面是一个使用vector <string>和map<string,string>实现字符串查找和替换的例子。
```cpp
#include <iostream>
#include <vector>
#include <map>
#include <string>
using namespace std;
// 字符串查找和替换函数
string findAndReplace(string str, const map<string,string>& replacements) {
for(const auto& replacement : replacements) {
size_t pos = str.find(replacement.first); // 查找要替换的字符串
while(pos != string::npos) {
str.replace(pos, replacement.first.length(), replacement.second); // 替换字符串
pos = str.find(replacement.first, pos + replacement.second.length()); // 继续查找下一个相同的字符串
}
}
return str;
}
int main() {
// 创建vector,存放网页每一行
vector<string> webPages = {
"<html>This is a website</html>",
"<body>Hello, world!</body>"
};
// 创建map,存放要替换的字符串
map<string, string> replacements = {
{"<html>", "<div>"},
{"</html>", "</div>"},
{"<body>", "<p>"},
{"</body>", "</p>"}
};
// 对每一行进行替换操作
for(auto& webPage : webPages) {
webPage = findAndReplace(webPage, replacements);
}
// 输出替换后的网页内容
for(const auto& webPage : webPages) {
cout << webPage << endl;
}
return 0;
}
```
运行以上代码,输出结果为:
```txt
<div>This is a website</div>
<p>Hello, world!</p>
```
以上代码中,首先定义了一个`findAndReplace`函数,该函数接受一个待处理的字符串和一个存放替换规则的map。函数通过遍历替换规则,使用`find`函数查找要替换的字符串,并使用`replace`函数进行替换。在每次替换后,继续查找下一个相同的字符串,直到不再找到为止。最后,返回替换完成的字符串。
在`main`函数中,我们创建了一个存放网页每一行的vector和一个存放替换规则的map。然后,对于每一行网页内容,我们调用`findAndReplace`函数进行替换操作。最后,将替换后的网页内容输出到控制台上。
阅读全文
相关推荐
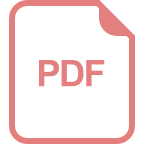
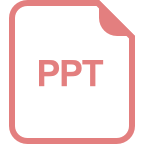
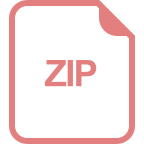
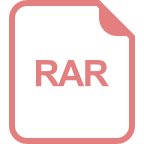
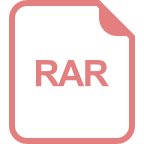
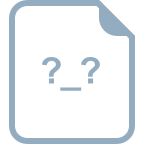
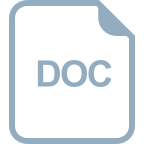
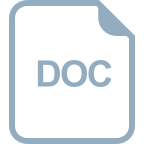








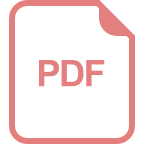
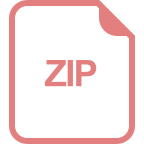