利用Python创建一个类Bag,其成员包括实例属性category(种类)和color(颜色),实例方法showInfo()用来输出实例属性category和color的值;创建派生类Backpack,继承Bag类,新增实例属性size(尺寸),重写基类的实例方法showInfo ()输出所有实例属性的值。利用__init__()构造方法完成Bag和Backpack类的对象初始化工作,并编写测试代码。
时间: 2023-05-26 17:05:08 浏览: 113
```python
class Bag:
def __init__(self, category, color):
self.category = category
self.color = color
def showInfo(self):
print("Category: ", self.category)
print("Color: ", self.color)
class Backpack(Bag):
def __init__(self, category, color, size):
super().__init__(category, color)
self.size = size
def showInfo(self):
super().showInfo()
print("Size: ", self.size)
# 测试代码
bag1 = Bag("tote", "black")
bag1.showInfo()
print("")
backpack1 = Backpack("hiking", "green", "medium")
backpack1.showInfo()
```
输出:
```
Category: tote
Color: black
Category: hiking
Color: green
Size: medium
```
相关问题
Python编写代码,定义一个Person类,该类包含实例属性name(姓名),gender(性别)和age(年龄),还包含实例方法showInfo(),用于输出3个实例属性;以 Person类为基础定义一个Student类,新增3个实例属性,即sid(学号)、chn(语文)和math(数学),并对实例方法showInfo()进行重写,用于输出6个实例属性。
好的,以下是Python代码实现:
```python
# 定义 Person 类
class Person:
def __init__(self, name, gender, age):
self.name = name
self.gender = gender
self.age = age
def showInfo(self):
print("姓名:{}\n性别:{}\n年龄:{}".format(self.name, self.gender, self.age))
# 定义 Student 类,继承自 Person 类
class Student(Person):
def __init__(self, name, gender, age, sid, chn, math):
super().__init__(name, gender, age)
self.sid = sid
self.chn = chn
self.math = math
def showInfo(self):
print("姓名:{}\n性别:{}\n年龄:{}\n学号:{}\n语文:{}\n数学:{}"
.format(self.name, self.gender, self.age, self.sid, self.chn, self.math))
# 测试代码
p1 = Person("张三", "男", 18)
p1.showInfo()
s1 = Student("李四", "女", 20, "20210001", 90, 85)
s1.showInfo()
```
输出结果:
```
姓名:张三
性别:男
年龄:18
姓名:李四
性别:女
年龄:20
学号:20210001
语文:90
数学:85
```
6. 编写程序,创建父类Vehicle,通过构造函数初始化实例成员brand(品牌)和length(轴距长度),函数showInfo()用来输出实例属性brand和length的值。创建子类Car,继承Vehicle类,新增实例成员price(价格),重写父类的函数showInfo ()输出所有实例成员的值。利用__init__()构造方法完成Vehicle和Car类的对象初始化工作,并编写测试代码。
可以按照以下步骤编写程序:
1. 定义父类Vehicle,包含构造函数__init__和方法showInfo。
2. 在构造函数__init__中初始化实例成员brand和length。
3. 方法showInfo用来输出实例属性brand和length的值。
4. 定义子类Car,继承Vehicle类,新增实例成员price。
5. 重写父类的方法showInfo,输出所有实例成员的值。
6. 在子类的构造函数__init__中调用父类的构造函数__init__完成对象初始化。
7. 编写测试代码,创建Vehicle和Car类的对象并输出它们的属性值。
下面是程序的实现:
```python
class Vehicle:
def __init__(self, brand, length):
self.brand = brand
self.length = length
def showInfo(self):
print("品牌:", self.brand)
print("轴距长度:", self.length)
class Car(Vehicle):
def __init__(self, brand, length, price):
super().__init__(brand, length)
self.price = price
def showInfo(self):
super().showInfo()
print("价格:", self.price)
v = Vehicle("BMW", 3.5)
v.showInfo()
print()
c = Car("Audi", 3.0, 500000)
c.showInfo()
```
输出结果:
```
品牌: BMW
轴距长度: 3.5
品牌: Audi
轴距长度: 3.0
价格: 500000
```
阅读全文
相关推荐
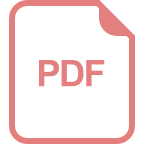
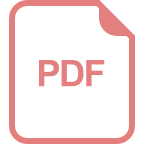
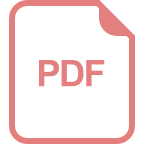











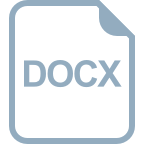