运用c++的知识,设计一个圆类 circle和一个桌子类 table,另设计一个圆桌类roundtable,它是从前两个类派生出来的。要求输出一个圆桌的高度,面积与颜色等。
时间: 2024-12-06 20:26:09 浏览: 19
在C++中,你可以创建如下的类结构来实现这个需求:
```cpp
class Circle {
public:
Circle(double radius, std::string color) : radius(radius), color(color) {}
double getArea() const { return M_PI * radius * radius; }
std::string getColor() const { return color; }
private:
double radius;
std::string color;
};
class Table {
public:
virtual ~Table() {}
virtual double getHeight() const = 0; // 虚函数,需要在派生类中实现
};
class RoundTable : public Table {
public:
RoundTable(double radius, double height, std::string color) : Circle(radius, color), height(height) {}
double getHeight() const override { return height; } // 实现父类的虚函数
double getArea() const override { return Circle::getArea(); } // 重写父类的纯虚函数
private:
double height;
};
int main() {
RoundTable roundTable(50.0, 75.0, "brown");
std::cout << "Round table height: " << roundTable.getHeight() << std::endl;
std::cout << "Round table area: " << roundTable.getArea() << std::endl;
std::cout << "Color of the round table: " << roundTable.getColor() << std::endl;
return 0;
}
```
在这个例子中,`Circle`表示圆形,`Table`是一个抽象基类,规定了有高度这个属性但没有提供获取方法。`RoundTable`从`Circle`派生,并实现了`Table`的`getHeight()`和`getArea()`方法,同时增加了自己的高度属性。
运行这段程序,你会得到圆桌的高度、面积以及颜色信息。
阅读全文
相关推荐
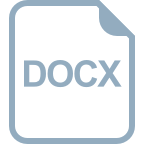
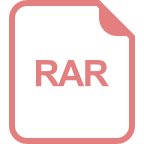
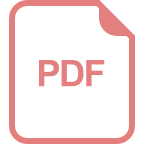






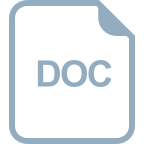
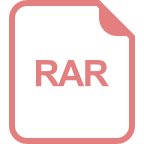
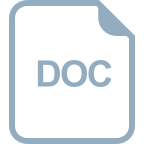
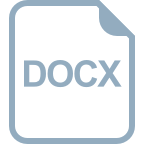
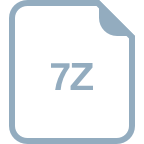
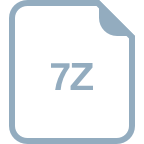
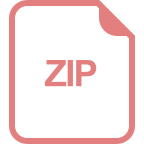