用c语言写一个二层通信代码
时间: 2024-01-29 21:02:15 浏览: 24
以下是一个简单的使用 C 语言实现的二层通信代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <net/ethernet.h>
#include <netinet/if_ether.h>
#include <netinet/in.h>
#include <unistd.h>
#define BUFFER_SIZE 65536
int main(int argc, char *argv[]) {
int sock, length;
struct sockaddr_in server_addr;
char buffer[BUFFER_SIZE];
// 创建原始套接字
sock = socket(AF_PACKET, SOCK_RAW, htons(ETH_P_ALL));
if (sock < 0) {
perror("socket() failed");
exit(EXIT_FAILURE);
}
// 绑定网卡
struct ifreq ifr;
memset(&ifr, 0, sizeof(ifr));
snprintf(ifr.ifr_name, sizeof(ifr.ifr_name), "%s", "eth0");
if (ioctl(sock, SIOCGIFINDEX, &ifr) < 0) {
perror("ioctl() failed");
close(sock);
exit(EXIT_FAILURE);
}
struct sockaddr_ll sll;
memset(&sll, 0, sizeof(sll));
sll.sll_family = AF_PACKET;
sll.sll_ifindex = ifr.ifr_ifindex;
sll.sll_protocol = htons(ETH_P_ALL);
if (bind(sock, (struct sockaddr*) &sll, sizeof(sll)) < 0) {
perror("bind() failed");
close(sock);
exit(EXIT_FAILURE);
}
// 接收数据帧并打印 MAC 地址
while (1) {
length = recv(sock, buffer, BUFFER_SIZE, 0);
if (length < 0) {
perror("recv() failed");
close(sock);
exit(EXIT_FAILURE);
}
struct ether_header *eth_hdr = (struct ether_header *) buffer;
printf("Source: %02x:%02x:%02x:%02x:%02x:%02x\n",
eth_hdr->ether_shost[0], eth_hdr->ether_shost[1],
eth_hdr->ether_shost[2], eth_hdr->ether_shost[3],
eth_hdr->ether_shost[4], eth_hdr->ether_shost[5]);
printf("Destination: %02x:%02x:%02x:%02x:%02x:%02x\n",
eth_hdr->ether_dhost[0], eth_hdr->ether_dhost[1],
eth_hdr->ether_dhost[2], eth_hdr->ether_dhost[3],
eth_hdr->ether_dhost[4], eth_hdr->ether_dhost[5]);
}
close(sock);
return 0;
}
```
这个程序使用了 Linux 的原始套接字来接收二层数据帧,并打印出源 MAC 地址和目的 MAC 地址。需要注意的是,这个程序需要 root 权限才能运行,并且需要修改 `eth0` 为你的网卡名称。
相关推荐
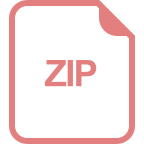
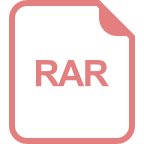
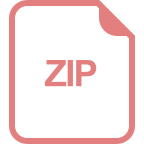














