flutter ios methodchannel
时间: 2023-10-27 18:03:05 浏览: 74
Flutter的MethodChannel是一种在Flutter和原生平台(如iOS)之间进行通信的机制。它允许Flutter应用程序调用原生平台上的方法,并且还可以让原生平台调用Flutter的方法。
在iOS上使用MethodChannel,首先需要在原生平台(Objective-C或Swift)的代码中创建一个MethodChannel实例。这个实例需要一个唯一的通道名称,以便Flutter可以识别它。然后,可以使用该MethodChannel实例来注册方法,并指定一个方法名称和一个回调函数来处理该方法被调用时的逻辑。
在Flutter应用程序中,可以使用MethodChannel的实例来调用原生平台上注册的方法。可以指定方法名称和传递参数(如果需要)。MethodChannel会将这个方法调用发送到原生平台,并等待原生平台返回结果。一旦结果返回,可以在Flutter端处理它。
使用MethodChannel时需要注意一些事项。首先,MethodChannel只能传递符合平台限制的数据类型,如字符串、数字、布尔值等。如果需要传递复杂的数据结构,可以将数据转换为平台能够识别的格式(如JSON),然后再进行传递。其次,MethodChannel是一种异步通信机制,所以在处理方法调用时需要考虑异步操作和结果处理的情况。
总的来说,Flutter的MethodChannel是一种强大而灵活的机制,可以实现Flutter应用程序与原生平台之间的双向通信。它为开发人员提供了在Flutter和iOS之间传递方法调用和数据的能力,可以实现更高级的功能和交互体验。
相关问题
flutter iOS版本更新
要更新Flutter iOS版本,可以按照以下步骤进行操作:
1. 更新Flutter SDK:首先需要更新Flutter SDK到最新版本。可以使用以下命令获取最新版本:
```bash
flutter upgrade
```
2. 更新Flutter插件:在Flutter项目中使用的插件可能需要更新到与Flutter SDK版本兼容的最新版本。可以使用以下命令更新Flutter插件:
```bash
flutter packages upgrade
```
3. 更新iOS依赖项:iOS依赖项也需要更新到与Flutter SDK版本兼容的最新版本。可以使用以下命令更新iOS依赖项:
```bash
cd ios
pod update
```
4. 清理和构建Flutter项目:更新完Flutter SDK、插件和iOS依赖项后,需要清理和构建Flutter项目,以确保更新生效。可以使用以下命令清理和构建Flutter项目:
```bash
flutter clean
flutter build ios
```
5. 在Xcode中更新项目:最后,在Xcode中打开项目,更新项目设置和依赖项。可以使用以下步骤在Xcode中更新项目:
- 打开Xcode
- 在菜单栏中选择 File -> Open,选择Flutter项目的iOS目录(通常在`/path/to/your/flutter/project/ios`)
- 在Xcode中选择项目文件,更新项目设置和依赖项
完成以上步骤后,就可以成功更新Flutter iOS版本了。
flutter ios 抓包
要在Flutter应用程序中捕获iOS设备上的网络流量,可以使用代理工具,例如Charles或Fiddler。以下是步骤:
1. 下载并安装Charles或Fiddler。
2. 在iOS设备上启用代理。打开“设置”->“无线局域网”->“当前连接的WiFi网络”->“配置代理”->“手动”,输入代理服务器的IP地址和端口号。
3. 在Charles或Fiddler上设置代理。在Charles中,打开“Proxy”->“Proxy Settings”,输入代理服务器的IP地址和端口号。在Fiddler中,打开“Tools”->“Options”->“Connections”,启用“Allow remote computers to connect”。
4. 运行Flutter应用程序,并确保设备与代理服务器连接。在Charles或Fiddler中,应该能够看到应用程序发出的网络请求和响应。
请注意,如果应用程序使用了HTTPS协议,代理服务器需要安装证书并进行配置,以便正常捕获流量。
相关推荐
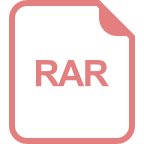
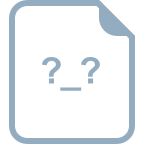
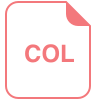











