帮我写一个Java快速排序
时间: 2023-09-22 13:05:47 浏览: 147
### 回答1:
对于Java快速排序,可以使用下面的代码:
public class QuickSort {
public static void sort(int[] array) {
quickSort(array, 0, array.length - 1);
}
private static void quickSort(int[] array, int start, int end) {
if (start >= end) {
return;
}
int pivotIndex = partition(array, start, end);
quickSort(array, start, pivotIndex - 1);
quickSort(array, pivotIndex + 1, end);
}
private static int partition(int[] array, int start, int end) {
int pivot = array[end];
int i = start;
for (int j = start; j < end; j++) {
if (array[j] <= pivot) {
swap(array, i, j);
i++;
}
}
swap(array, i, end);
return i;
}
private static void swap(int[] array, int i, int j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
### 回答2:
以下是一个使用Java实现的快速排序算法示例:
```java
public class QuickSort {
public static void quickSort(int[] array, int left, int right) {
if (left < right) {
int pivot = partition(array, left, right);
quickSort(array, left, pivot - 1);
quickSort(array, pivot + 1, right);
}
}
private static int partition(int[] array, int left, int right) {
int pivot = array[right];
int i = left - 1;
for (int j = left; j < right; j++) {
if (array[j] <= pivot) {
i++;
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
int temp = array[i + 1];
array[i + 1] = array[right];
array[right] = temp;
return i + 1;
}
public static void main(String[] args) {
int[] array = {6, 3, 8, 9, 1, 2, 4, 7, 5};
int length = array.length;
System.out.println("原始数组:");
for (int num : array) {
System.out.print(num + " ");
}
quickSort(array, 0, length - 1);
System.out.println("\n排序后的数组:");
for (int num : array) {
System.out.print(num + " ");
}
}
}
```
快速排序是一种常用的排序算法,其基本思想是通过在数组中选择一个基准元素,将数组划分为两部分,一部分小于基准元素,一部分大于基准元素,然后递归地对两部分进行排序。
在上述代码中,`quickSort`方法接收一个数组、左边界和右边界作为参数,并使用`partition`方法将数组划分为两部分。`partition`方法选择数组最后一个元素作为基准元素,然后遍历数组并将小于等于基准元素的元素交换到数组左边,并返回基准元素的索引。
通过不断递归调用`quickSort`方法,在每次划分中将数组分成两个子数组,直到左边界小于右边界为止。最后,输出排序后的数组。
以上就是一个基本的Java快速排序实现。快速排序的平均时间复杂度为O(nlogn),是一种高效的排序算法。
### 回答3:
快速排序是一种常用的排序算法,它的基本思想是通过不断地将数列分割为较小和较大的两部分,然后递归地在两部分继续进行快速排序,最终完成排序。
下面是一个Java实现的快速排序算法:
```java
public class QuickSort {
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivotIndex = partition(arr, low, high);
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[low];
int i = low + 1;
int j = high;
while (i <= j) {
while (i <= j && arr[i] <= pivot) {
i++;
}
while (i <= j && arr[j] > pivot) {
j--;
}
if (i < j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int temp = arr[j];
arr[j] = arr[low];
arr[low] = temp;
return j;
}
public static void main(String[] args) {
int[] arr = {5, 2, 9, 1, 6, 8, 3};
quickSort(arr, 0, arr.length - 1);
System.out.println("排序结果:");
for (int num : arr) {
System.out.print(num + " ");
}
}
}
```
以上就是一个Java实现的快速排序算法。我们先定义了一个`quickSort`方法,用于进行快速排序。在`quickSort`方法内部,我们通过`partition`方法将数列分为较小和较大的两部分,并分别对这两部分再调用`quickSort`方法进行递归排序,最终完成排序。
`partition`方法定义了一个基准值`pivot`,通过两个指针`i`和`j`分别从左右两边向中间扫描,找到需要交换的元素后进行交换。最后将基准值放到正确的位置上,然后返回该位置作为分割点。
在`main`方法中,我们定义了一个测试数组`arr`,并调用`quickSort`方法进行排序。排序完成后,输出排序结果。
希望以上的解答对您有帮助,如果有任何问题,请随时提问。
阅读全文
相关推荐







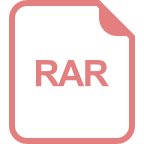



