这是functions.py文件,记录要用到的函数
时间: 2024-02-13 19:05:28 浏览: 53
好的,我看一下。
```python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.colors import ListedColormap
from sklearn.datasets import make_moons
from sklearn.model_selection import train_test_split
def sigmoid(x):
return 1 / (1 + np.exp(-x))
def relu(x):
return np.maximum(0, x)
def softmax(x):
exp_x = np.exp(x)
return exp_x / np.sum(exp_x, axis=1, keepdims=True)
def cross_entropy(y_pred, y_true):
m = y_pred.shape[0]
p = softmax(y_pred)
log_likelihood = -np.log(p[range(m), y_true])
loss = np.sum(log_likelihood) / m
return loss
def plot_decision_boundary(model, X, y):
cmap = ListedColormap(['#FF0000', '#00FF00', '#0000FF'])
h = 0.02
x_min, x_max = X[:, 0].min() - 0.5, X[:, 0].max() + 0.5
y_min, y_max = X[:, 1].min() - 0.5, X[:, 1].max() + 0.5
xx, yy = np.meshgrid(np.arange(x_min, x_max, h), np.arange(y_min, y_max, h))
Z = model(np.c_[xx.ravel(), yy.ravel()])
Z = np.argmax(Z, axis=1)
Z = Z.reshape(xx.shape)
plt.contourf(xx, yy, Z, cmap=cmap)
plt.scatter(X[:, 0], X[:, 1], c=y, cmap=cmap)
plt.show()
def load_dataset():
X, y = make_moons(n_samples=1000, noise=0.2, random_state=100)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
return X_train, X_test, y_train, y_test
```
这个文件中定义了一些神经网络常用的函数,如sigmoid、relu、softmax、交叉熵损失函数等。还有一个绘制决策边界的函数`plot_decision_boundary`和一个生成数据集的函数`load_dataset`。这些函数都是比较常用的,看起来没有问题。
阅读全文
相关推荐
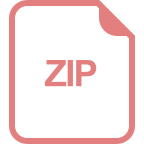
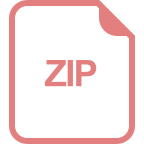
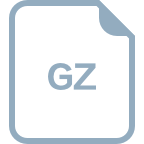
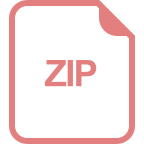
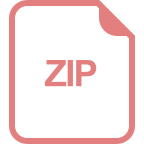
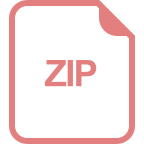
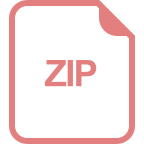
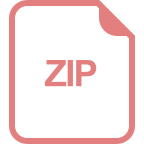
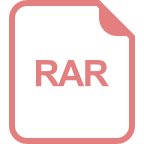
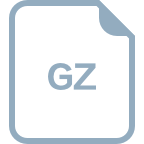
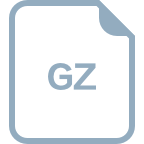
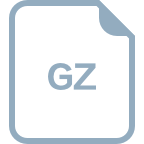
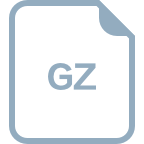
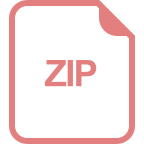
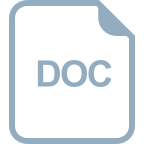
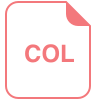
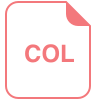