QTimer异步执行一个函数
时间: 2024-03-28 19:42:06 浏览: 16
可以使用QTimer的singleShot方法来实现异步执行一个函数。具体步骤如下:
1. 定义一个函数,例如:
```
def my_func():
print("执行函数")
```
2. 使用QTimer的singleShot方法,设置定时器触发时执行的函数,例如:
```
from PyQt5.QtWidgets import QApplication
from PyQt5.QtCore import QTimer
app = QApplication([])
timer = QTimer()
timer.timeout.connect(my_func)
timer.setSingleShot(True)
timer.start(1000)
app.exec_()
```
上述代码中,设置定时器的间隔为1000毫秒,即1秒钟,定时器触发时会执行my_func函数。由于设置了setSingleShot(True),所以定时器只会触发一次。最后调用app.exec_()启动事件循环,保证程序不会退出。
相关问题
pyqt6 如何异步执行函数
在 PyQt6 中,可以使用 `QTimer.singleShot` 方法来实现异步执行函数的效果。这个方法允许您在指定的时间间隔之后执行一个函数。
下面是一个示例,演示了如何使用 `QTimer.singleShot` 实现异步执行函数:
```python
from PyQt6.QtCore import QTimer
# 定义一个需要异步执行的函数
def my_async_function():
print("Async function executed")
# 创建一个 QTimer 对象
timer = QTimer()
# 定义一个槽函数,用于执行异步函数
def execute_async_function():
my_async_function()
# 停止定时器
timer.stop()
# 将槽函数与定时器的 timeout 信号关联
timer.timeout.connect(execute_async_function)
# 启动定时器,设置延迟时间为0毫秒
timer.singleShot(0)
# 执行其他操作
print("Other operations")
```
在上面的示例中,`my_async_function` 是需要异步执行的函数。使用 `QTimer.singleShot` 设置定时器的延迟时间为0毫秒,这样定时器会立即触发。
然后,定时器触发后,会执行 `execute_async_function` 槽函数,该槽函数会调用 `my_async_function` 函数。最后,定时器会被停止。
注意,在执行异步函数之后,程序会继续执行其他操作。在上面的示例中,会先打印 "Async function executed",然后再打印 "Other operations"。
这样,您就可以在 PyQt6 中实现异步执行函数的效果了。
QTimer::singleShot 延时0s执行doSomething函数
虽然你使用了QTimer::singleShot函数,但是它的本意是在延迟一定时间后执行指定函数。如果你想要立即执行doSomething函数,可以直接调用它,而不需要使用QTimer::singleShot函数。例如:
```
doSomething();
```
如果你想要延迟一定时间后执行doSomething函数,可以这样使用QTimer::singleShot函数:
```
QTimer::singleShot(0, this, &MyClass::doSomething);
```
这里的0表示延迟0毫秒,即立即执行。但是因为QTimer::singleShot函数是异步执行的,所以它并不会立即执行doSomething函数,而是会将doSomething函数的执行放到事件循环中,等待下一个事件循环时执行。
相关推荐











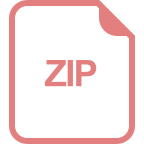