c# Cognitive Services
时间: 2024-05-25 18:13:21 浏览: 150
Cognitive Services 是微软提供的一组人工智能服务,其中包括语音识别、图像分析、自然语言处理等多个方面的功能。C# 是一种面向对象的编程语言,C# 开发人员可以使用 Cognitive Services 提供的 API 来实现人工智能应用,例如语音识别、情感分析、文本翻译等。Cognitive Services 中的 API 都支持 REST 和 SOAP 协议,因此可以在 C# 中使用 HttpClient、WebRequest 等类来发起 API 请求。微软还提供了 C# 的 SDK,使得 C# 开发人员更方便地使用 Cognitive Services。
相关问题
C#人脸活体检测
C#中实现人脸活体检测可以使用Microsoft的Cognitive Services中的Face API。Face API提供了现代化的人脸检测和识别功能,包括活体检测。使用Face API,您可以检测到面部表情和动作,并验证用户是否是真人而不是照片或视频。
下面是实现人脸活体检测的简单步骤:
1. 注册Microsoft Cognitive Services账户并创建一个Face API服务。
2. 安装Microsoft.Azure.CognitiveServices.Vision.Face NuGet包。
3. 使用Face API进行人脸检测和活体检测,示例代码如下:
```csharp
using Microsoft.Azure.CognitiveServices.Vision.Face;
using Microsoft.Azure.CognitiveServices.Vision.Face.Models;
public async Task<bool> DetectLiveFace(string imageFilePath)
{
var faceClient = new FaceClient(new ApiKeyServiceClientCredentials("YOUR_FACE_API_KEY"));
faceClient.Endpoint = "YOUR_FACE_API_ENDPOINT";
using (Stream imageFileStream = File.OpenRead(imageFilePath))
{
IList<DetectedFace> faces = await faceClient.Face.DetectWithStreamAsync(imageFileStream, true, false, new List<FaceAttributeType> { FaceAttributeType.HeadPose, FaceAttributeType.Emotion });
foreach (var face in faces)
{
var headPose = face.FaceAttributes.HeadPose;
var emotion = face.FaceAttributes.Emotion;
if (headPose.Pitch > 20 || headPose.Roll > 20 || emotion.Neutral > 0.5)
{
return false;
}
}
}
return true;
}
```
在这个示例中,我们使用Face API的DetectWithStreamAsync方法检测人脸,并使用FaceAttributes属性获取人脸的头部姿势和情绪。如果头部姿势超过20度或情感中的“中性”分数超过0.5,则认为该面部不是真人面部。
Himage转ICogImage的C#程序
Himage和ICogImage都是特定于某个软件或应用的数据结构,通常与某些图像处理或人工智能平台有关。然而,由于缺乏上下文,我无法提供具体的C#转换代码,因为这依赖于它们的实际含义和对应的库或框架。
如果Himage是来自某个自定义库或者某个特定应用的类型,而ICogImage是来自 Cognitive Services (如Microsoft Azure Computer Vision) 或其SDK,那么转换代码可能会像下面这样:
```csharp
using Microsoft.Azure.CognitiveServices.Vision.ComputerVision;
using System.IO;
// 假设Himage是你自定义的数据类型,ICogImage是Azure Cognitive Services的类型
public ICogImage ConvertToCogImage(Himage hImage)
{
// 创建一个ComputerVisionClient实例
var client = new ComputerVisionClient(new ApiKeyServiceClientCredentials("<your_api_key>"))
{
Endpoint = "<your_endpoint>.cognitiveservices.azure.com"
};
using (var imageStream = new MemoryStream())
{
// 将Himage数据写入内存流
hImage.Save(imageStream); // 这里假设Himage有自己的Save方法将数据保存到流
// 使用client的RecognizeGenericEntitiesAsync方法,这里只是示例,实际转换会根据需要调整
var result = client.RecognizeGenericEntitiesInStreamAsync(imageStream).Result;
// 返回转换后的ICogImage对象,例如从识别结果中获取并返回相关图像信息
return result; // 实际上应该是一个包含所需属性的对象
}
}
// 调用函数
ICogImage cogImage = ConvertToCogImage(yourHimageInstance);
```
阅读全文
相关推荐
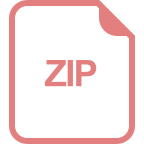
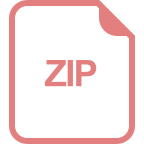
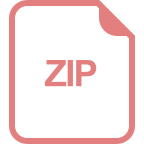









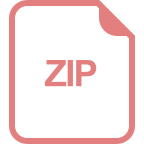
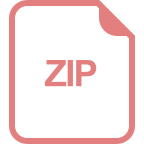
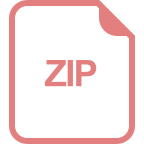
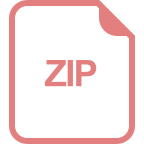
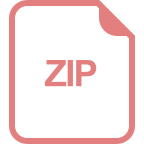