编程小学数学学习系统, 具有随机出题、自动评分、查看正确答案、错题重做等功能的测试系统。山 设计程序运行界面 用户登录功能一 能用菜单选择测试系统的各功能,写出代码
时间: 2024-03-20 18:43:44 浏览: 32
以下是一个简单的 Python 实现,实现了基本的功能,可以根据实际需求进行改进:
```python
import random
# 存储用户信息
users = {}
# 生成随机题目
def generate_question():
# 题目类型:加、减、乘、除
operators = ['+', '-', '*', '/']
operator = random.choice(operators)
# 生成两个操作数
if operator == '+':
a = random.randint(1, 100)
b = random.randint(1, 100)
elif operator == '-':
a = random.randint(1, 100)
b = random.randint(1, a)
elif operator == '*':
a = random.randint(1, 10)
b = random.randint(1, 10)
else:
b = random.randint(1, 10)
a = b * random.randint(1, 10)
# 生成题目字符串
question = str(a) + ' ' + operator + ' ' + str(b) + ' = ?'
# 计算正确答案
if operator == '/':
answer = a // b
else:
answer = eval(str(a) + operator + str(b))
return question, answer
# 用户登录
def login():
username = input('请输入用户名:')
if username in users:
print('欢迎回来,', username)
else:
users[username] = {'score': 0, 'questions': []}
print('欢迎新用户', username)
# 生成试卷
def generate_paper():
paper = []
for i in range(10):
question, answer = generate_question()
paper.append((question, answer))
return paper
# 开始考试
def start_exam():
paper = generate_paper()
score = 0
for i, (question, answer) in enumerate(paper):
print('第{}题:{}'.format(i+1, question))
user_answer = input('请输入答案:')
if int(user_answer) == answer:
print('回答正确!')
score += 10
else:
print('回答错误,正确答案是', answer)
users[username]['questions'].append((question, answer, user_answer))
users[username]['score'] += score
print('考试结束,本次得分:', score)
# 查看历史成绩
def view_score():
if 'score' not in users[username]:
print('您还没有进行过考试')
return
score = users[username]['score']
print('您的历史成绩为:', score)
# 查看错题
def view_mistakes():
if 'questions' not in users[username]:
print('您还没有进行过考试')
return
mistakes = [(q, a, ua) for q, a, ua in users[username]['questions'] if int(ua) != a]
if not mistakes:
print('恭喜您,没有错题!')
else:
print('您的错题如下:')
for q, a, ua in mistakes:
print('题目:', q)
print('您的答案:', ua)
print('正确答案:', a)
# 主程序
while True:
print('欢迎使用小学数学学习系统')
print('请选择要进行的操作:')
print('1. 登录')
print('2. 开始考试')
print('3. 查看历史成绩')
print('4. 查看错题')
print('5. 退出')
choice = input()
if choice == '1':
login()
elif choice == '2':
if 'score' not in users[username]:
print('您还没有进行过考试')
continue
start_exam()
elif choice == '3':
view_score()
elif choice == '4':
view_mistakes()
elif choice == '5':
print('谢谢使用,再见!')
break
```
相关推荐
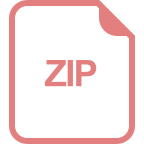
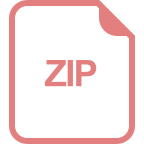
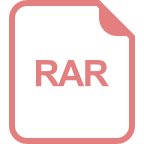






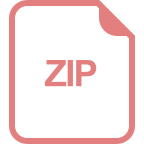
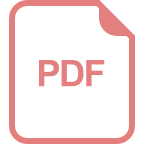
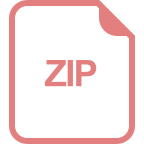
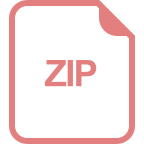
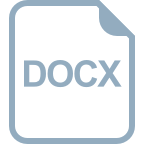
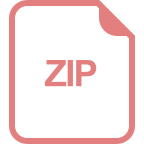
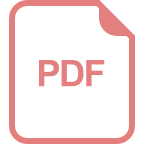