选择一幅图像,对其进行Canny 边缘检测
时间: 2024-09-28 14:15:40 浏览: 47
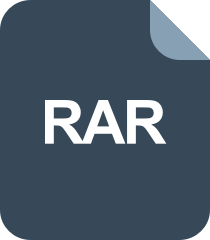
图像的边缘检测(程序有GUI)
Canny边缘检测是一种常用的图像处理技术,它由John F. Canny于1986年提出,用于找出图像中的强边缘。以下是使用Python和OpenCV库进行Canny边缘检测的基本步骤,假设我们已经有一幅名为"example.jpg"的图像:
```python
import cv2
import numpy as np
# 1. 读取图像
img = cv2.imread('example.jpg', cv2.IMREAD_GRAYSCALE)
# 2. 高斯滤波 - 减少噪声影响
blurred_img = cv2.GaussianBlur(img, (5, 5), 0)
# 3. 计算梯度幅值和方向
sobel_x = cv2.Sobel(blurred_img, cv2.CV_64F, 1, 0, ksize=3)
sobel_y = cv2.Sobel(blurred_img, cv2.CV_64F, 0, 1, ksize=3)
gradient_magnitude = np.sqrt(sobel_x**2 + sobel_y**2)
gradient_angle = np.arctan2(sobel_y, sobel_x)
# 4. 非极大值抑制(Non-Maximum Suppression)
angle_gradients = np.hypot(sobel_x, sobel_y)
directions = np.vstack((np.cos(gradient_angle), np.sin(gradient_angle)))
indices = np.argmax(angle_gradients, axis=0)
angles = directions[indices]
canny_output = np.zeros_like(angle_gradients)
canny_output[np.arange(len(angles)), angles.astype(int)] = angle_gradients
# 5. 双阈值处理(Double Thresholding)
low_threshold, high_threshold = 50, 150 # 可调整阈值
edges = np.zeros_like(canny_output)
edges[(canny_output >= low_threshold) & (canny_output <= high_threshold)] = 255
# 6. 寻找连续区域
edges = cv2.Canny(img, low_threshold, high_threshold)
# 显示原始图像及结果
cv2.imshow("Original Image", img)
cv2.imshow("Canny Edges", edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个过程中,我们先模糊图像减少噪声,然后计算梯度及其方向,接着进行非极大值抑制和双阈值处理,最后连接边缘形成最终的Canny边框。
阅读全文
相关推荐
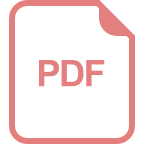
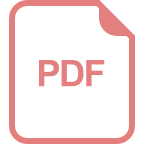















