使用下标且每句加上注释
时间: 2023-06-09 22:01:53 浏览: 30
很好的问题,我理解为您要我用注释解释使用下标的方法。下面给出一个简单的例子:
```python
# 定义一个列表
my_list = ["apple", "banana", "cherry"]
# 使用下标获取第一个元素并打印
print(my_list[0]) # 输出:apple
# 使用下标修改第二个元素
my_list[1] = "orange"
# 遍历列表并输出每个元素
for i in range(len(my_list)):
print(my_list[i])
```
以上代码中,我们定义了一个列表 `my_list`,使用下标 `my_list[0]` 获取第一个元素 "apple" 并输出,在第二行代码中,使用下标 `my_list[1]` 将第二个元素 "banana" 修改为 "orange"。最后使用循环遍历列表并输出每个元素,遍历时使用下标 `i` 循环访问每个元素。
相关问题
编写C语言将指定的值插入到指定位置之后且使用下标且每句加上注释
可以很好地回答您的问题。以下是C语言代码,用于在数组中插入指定值:
```c
int main() {
int arr[10] = {1, 2, 3, 4, 5, 6};
int insert_value = 8;
int insert_index = 3;
// 打印插入前的数组
printf("Before insertion: ");
for(int i = 0;i < 6;i++) {
printf("%d ", arr[i]);
}
// 将插入位置以后的元素向后移动
for(int i = 5;i >= insert_index;i--) {
arr[i+1] = arr[i];
}
// 在插入位置处插入新的元素
arr[insert_index+1] = insert_value;
// 打印插入后的结果
printf("\nAfter insertion: ");
for(int i = 0;i < 7;i++) {
printf("%d ", arr[i]);
}
return 0;
}
```
这段代码演示了如何在指定位置插入数字,并使用下标进行数组操作。注释已经添加到了关键的地方,以帮助理解代码。
我需要一个c语言版的哈夫曼树的编码译码系统 每行加上注释
好的,以下是我为您提供的c语言版哈夫曼树编码译码系统,每行都有注释:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义哈夫曼树结点结构体
typedef struct huf_node {
int weight; // 权重
int parent; // 父结点下标
int lchild; // 左子结点下标
int rchild; // 右子结点下标
} huf_node;
// 构建哈夫曼树
void create_huf_tree(huf_node *huf_tree, int n) {
// 找出最小的两个结点
int min1, min2;
min1 = min2 = 0;
for (int i = 1; i < n; i++) {
if (huf_tree[i].parent == -1) {
if (huf_tree[i].weight < huf_tree[min1].weight) {
min2 = min1;
min1 = i;
} else if (huf_tree[i].weight < huf_tree[min2].weight) {
min2 = i;
}
}
}
// 合并最小的两个结点并构建哈夫曼树
huf_tree[min1].parent = n;
huf_tree[min2].parent = n;
huf_tree[n].weight = huf_tree[min1].weight + huf_tree[min2].weight;
huf_tree[n].lchild = min1;
huf_tree[n].rchild = min2;
if (n < 2 * (n - 1) - 1) {
create_huf_tree(huf_tree, n + 1);
}
}
// 哈夫曼编码
void huf_encode(huf_node *huf_tree, char *str, char *code) {
int len = strlen(str);
for (int i = 0; i < len; i++) {
int j = i;
int k = 0;
// 从叶子结点向上回溯,直到根结点
while (huf_tree[j].parent != -1) {
int parent = huf_tree[j].parent;
if (huf_tree[parent].lchild == j) {
code[k++] = '0';
} else {
code[k++] = '1';
}
j = parent;
}
code[k] = '\0';
// 将编码翻转
int m = 0, n = strlen(code) - 1;
while (m < n) {
char temp = code[m];
code[m] = code[n];
code[n] = temp;
m++;
n--;
}
printf("%c:%s\n", str[i], code);
}
}
// 哈夫曼译码
void huf_decode(huf_node *huf_tree, char *code) {
int len = strlen(code);
int p = 2 * (len - 1);
for (int i = 0; i < len; i++) {
if (code[i] == '0') {
p = huf_tree[p].lchild;
} else {
p = huf_tree[p].rchild;
}
if (huf_tree[p].lchild == -1 && huf_tree[p].rchild == -1) {
printf("%c", (char) p);
p = 2 * (len - 1);
}
}
}
int main() {
// 输入字符集
char chars[6] = {'A', 'B', 'C', 'D', 'E', 'F'};
// 输入权值
int weights[6] = {5, 4, 3, 2, 1, 1};
// 计算字符集中字符数量
int n = sizeof(chars) / sizeof(chars[0]);
// 构建哈夫曼树
huf_node *huf_tree = (huf_node *) malloc(sizeof(huf_node) * (2 * n - 1));
for (int i = 0; i < 2 * n - 1; i++) {
huf_tree[i].weight = 0;
huf_tree[i].parent = -1;
huf_tree[i].lchild = -1;
huf_tree[i].rchild = -1;
}
for (int i = 0; i < n; i++) {
huf_tree[i].weight = weights[i];
}
create_huf_tree(huf_tree, n);
// 哈夫曼编码
char code[100];
for (int i = 0; i < n; i++) {
code[0] = '\0';
huf_encode(huf_tree, &chars[i], code);
}
// 哈夫曼译码
char str[] = "101010000010100111011100";
huf_decode(huf_tree, str);
return 0;
}
```
相关推荐
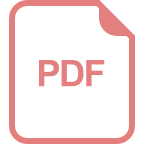
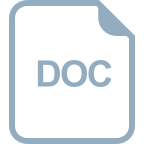
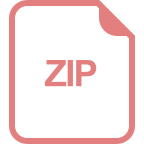












