python每秒随机获取10到30之间的一个浮点数,展出一个滚动的折线图,x轴刻度为时间,设置上限为20,下限为15,超过上限或者低于下限的值,改变颜色,每个数据点要有数值标识,pySimpleGUI建立一个界面,有开始,暂停,继续,退出,四个按钮来控制实时折线图
时间: 2024-05-09 16:14:51 浏览: 36
好的,我会尽力回答您的问题。首先,您需要使用Python中的random模块生成10到30之间的随机数。然后,您需要使用matplotlib库来绘制折线图。以下是一个简单的示例代码:
```python
import random
import matplotlib.pyplot as plt
import matplotlib.animation as animation
x_values = []
y_values = []
upper_limit = 20
lower_limit = 15
fig, ax = plt.subplots()
line, = ax.plot(x_values, y_values)
def update(num):
global x_values, y_values
x_values.append(num)
y = random.uniform(10, 30)
y_values.append(y)
line.set_data(x_values, y_values)
if y > upper_limit or y < lower_limit:
line.set_color('red')
else:
line.set_color('blue')
ax.relim()
ax.autoscale_view()
return line,
ani = animation.FuncAnimation(fig, update, frames=range(100), interval=100)
plt.show()
```
这段代码会生成一个实时折线图,并且会根据数据点的值改变线条的颜色。您可以根据需要修改上限、下限和间隔时间。接下来,您可以使用PySimpleGUI库创建一个GUI界面,添加开始、暂停、继续和退出按钮,并将matplotlib图形嵌入到窗口中。以下是示例代码:
```python
import PySimpleGUI as sg
import random
import matplotlib.pyplot as plt
import matplotlib.animation as animation
from matplotlib.backends.backend_tkagg import FigureCanvasTkAgg
x_values = []
y_values = []
upper_limit = 20
lower_limit = 15
fig, ax = plt.subplots()
line, = ax.plot(x_values, y_values)
def update(num):
global x_values, y_values
x_values.append(num)
y = random.uniform(10, 30)
y_values.append(y)
line.set_data(x_values, y_values)
if y > upper_limit or y < lower_limit:
line.set_color('red')
else:
line.set_color('blue')
ax.relim()
ax.autoscale_view()
return line,
ani = animation.FuncAnimation(fig, update, frames=range(100), interval=100)
canvas = FigureCanvasTkAgg(fig, master=sg.Window('Real-time Plot', [[sg.Canvas(key='-CANVAS-')], [sg.Button('Start'), sg.Button('Pause'), sg.Button('Resume'), sg.Button('Exit')]]))
canvas.draw()
canvas.get_tk_widget().pack(side='top', fill='both', expand=1)
sg.theme('DarkBlue')
while True:
event, values = sg.Window('Real-time Plot', [[sg.Canvas(key='-CANVAS-')], [sg.Button('Start'), sg.Button('Pause'), sg.Button('Resume'), sg.Button('Exit')]]).read(close=True)
if event == 'Start':
ani.event_source.start()
elif event == 'Pause':
ani.event_source.stop()
elif event == 'Resume':
ani.event_source.start()
elif event == 'Exit' or event == sg.WIN_CLOSED:
break
```
这段代码会生成一个带有开始、暂停、继续和退出按钮的GUI界面,并将matplotlib图形嵌入到窗口中。您可以根据需要修改按钮标签和GUI主题。
阅读全文
相关推荐
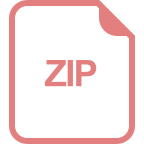
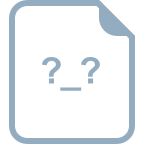

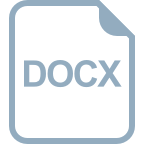
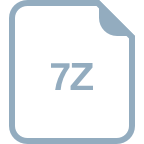
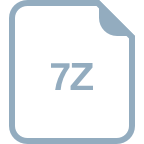
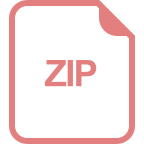
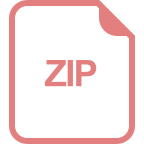
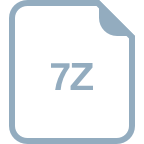
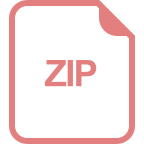
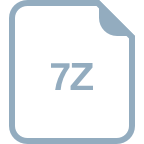
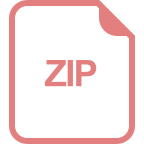