python .websocket 发送信息
时间: 2023-07-04 10:15:33 浏览: 160
要在 Python 中通过 websocket 发送消息,可以使用 `send` 方法。在 `on_open` 回调函数中,你可以调用 `ws.send` 来向服务器发送消息,例如:
```python
def on_open(ws):
print("### open ###")
ws.send("Hello, Server!")
```
这将向服务器发送一个字符串消息 "Hello, Server!"。当然,你可以根据需要修改消息内容。如果需要发送其他类型的数据,例如 JSON 对象,可以使用 `json.dumps` 方法将其转换为字符串,然后再发送,例如:
```python
import json
def on_open(ws):
print("### open ###")
message = {"type": "login", "username": "Alice", "password": "123456"}
ws.send(json.dumps(message))
```
这将向服务器发送一个 JSON 对象,包含了登录信息。
相关问题
python websocket发送信息
在Python中,你可以使用`websocket`模块来发送WebSocket消息。下面是一个简单的示例代码,演示如何发送WebSocket消息:
```python
import websocket
def on_message(ws, message):
print(f"Received message: {message}")
def on_error(ws, error):
print(f"Error: {error}")
def on_close(ws):
print("WebSocket connection closed")
def on_open(ws):
# 发送消息
ws.send("Hello, WebSocket!")
if __name__ == "__main__":
# 创建WebSocket连接
ws = websocket.WebSocketApp("ws://example.com/socket",
on_message=on_message,
on_error=on_error,
on_close=on_close)
ws.on_open = on_open
# 启动WebSocket连接
ws.run_forever()
```
在上面的示例中,你需要将`"ws://example.com/socket"`替换为实际的WebSocket服务器地址。通过调用`ws.send()`方法,你可以发送消息到WebSocket服务器。
请注意,你需要使用`websocket`模块来运行这个代码。你可以使用以下命令安装它:
```
pip install websocket-client
```
希望能帮助到你!如果有任何问题,请随时提出。
autobahn.twisted.websocket举例
autobahn.twisted.websocket是一个Python库,它提供了在Twisted框架下使用WebSocket的功能。使用autobahn.twisted.websocket,你可以轻松地创建WebSocket客户端和服务器,以实现实时通信。下面是一个简单的例子,演示如何使用autobahn.twisted.websocket创建一个WebSocket服务器:
```python
from twisted.internet import reactor
from autobahn.twisted.websocket import WebSocketServerFactory, \
WebSocketServerProtocol
class MyServerProtocol(WebSocketServerProtocol):
def onConnect(self, request):
print("Client connected: {0}".format(request.peer))
def onOpen(self):
print("WebSocket connection open.")
def onMessage(self, payload, isBinary):
if isBinary:
print("Binary message received: {0} bytes".format(len(payload)))
else:
print("Text message received: {0}".format(payload.decode('utf8')))
# echo message back to client
self.sendMessage(payload, isBinary)
def onClose(self, wasClean, code, reason):
print("WebSocket connection closed: {0}".format(reason))
if __name__ == '__main__':
factory = WebSocketServerFactory("ws://localhost:9000")
factory.protocol = MyServerProtocol
reactor.listenTCP(9000, factory)
reactor.run()
```
这个例子创建了一个WebSocket服务器,监听在本地的9000端口。当客户端连接时,会打印出相应的信息。当收到消息时,会将其原封不动地发送回客户端。当客户端断开连接时,同样会打印出相应的信息。
阅读全文
相关推荐
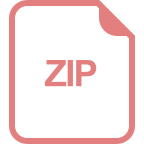
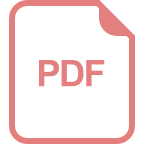
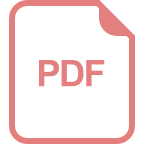
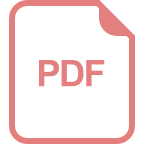
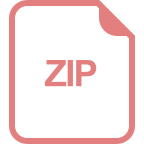
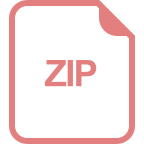
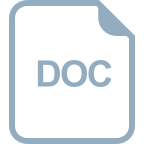
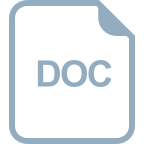
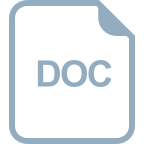
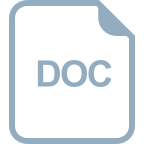
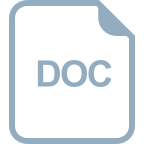
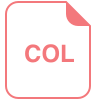
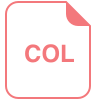

