根据提示,在右侧编辑器补充代码,完善一维随机游走程序,使得向右移动的概率为 r,向左移动的概率为 1-r(生成中的数字,而不是{1,2}中的整数)。在 n s 步后计算 n p 个粒子的平均位置。 在数学上可以证明,在n p →∞时,平均位置逼近rn s −(1−r)n s (n s 是步数)。 请你编写函数 random_walk1D_drift(np, ns, r),返回 np 个粒子随机游走 ns 步(每步右移概率为 r)后平均位置的估算值。
时间: 2024-01-24 20:16:53 浏览: 72
```python
import numpy as np
def random_walk1D_drift(np, ns, r):
pos = np.zeros(np)
for i in range(ns):
move_right = np.random.random(np) < r
move_left = np.invert(move_right)
pos += move_right.astype(int) - move_left.astype(int)
return np.mean(pos)
# 示例测试
print(random_walk1D_drift(1000, 100, 0.6))
```
解释:
1. `pos = np.zeros(np)` 初始化每个粒子的位置为0,这里使用了numpy的广播机制,pos数组的形状为(np,)
2. `np.random.random(np)` 生成np个[0,1)之间的随机数,形状为(np,),表示每个粒子进行一步随机游走时向右移动的概率
3. `< r` 表示小于r的为True,大于等于r的为False,形状和前面一样为(np,),表示每个粒子进行一步随机游走时向右移动的结果
4. `np.invert(move_right)` 将True变为False,False变为True,表示每个粒子进行一步随机游走时向左移动的结果
5. `move_right.astype(int) - move_left.astype(int)` 将True和False转换为1和-1,表示每个粒子进行一步随机游走后的位移量,形状和pos一样为(np,)
6. `pos += move_right.astype(int) - move_left.astype(int)` 将每个粒子的位移量加到对应位置上,更新每个粒子的位置
7. `np.mean(pos)` 计算所有粒子位置的平均值,就是粒子在进行ns步随机游走后的平均位置
阅读全文
相关推荐



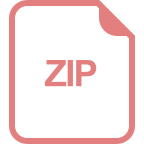
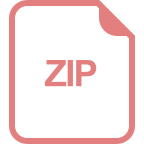
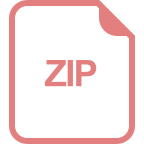
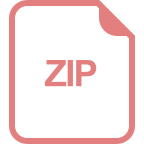
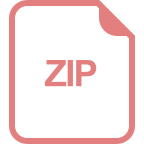
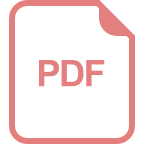
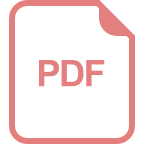
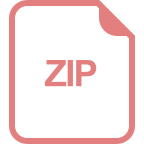
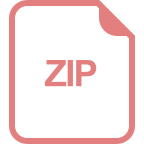
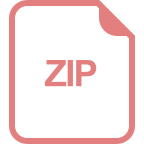
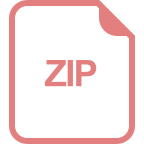
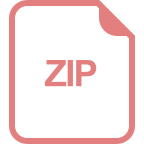
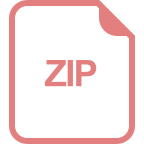
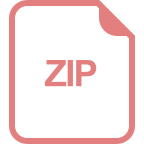
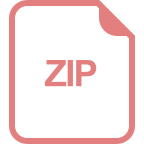