编写一段代码使用deeplabv3+网络实现遥感影像中建筑物的自动提取
时间: 2023-08-31 12:35:02 浏览: 131
### 回答1:
我们可以使用下面的代码来实现遥感影像中建筑物的自动提取:# 导入必要的库
from keras.applications.deeplabv3 import Deeplabv3
import numpy as np# 加载你的遥感图像
image = np.array(data)# 创建Deeplabv3模型实例
model = Deeplabv3(input_shape=(224, 224, 3), classes=1)# 运行模型
result = model.predict(image)# 解析结果,提取建筑物
buildings = result > 0.5
### 回答2:
要使用deeplabv3网络实现遥感影像中建筑物的自动提取,首先需要安装相关的深度学习框架和库,如TensorFlow和Keras。然后按照以下步骤编写代码:
1. 导入所需的库和模块:
```python
import numpy as np
from PIL import Image
import tensorflow as tf
from tensorflow.keras.preprocessing import image
from tensorflow.keras.applications.deeplab_v3 import preprocess_input
```
2. 加载预训练的deeplabv3模型:
```python
model = tf.keras.applications.DeepLabV3(weights='cityscapes')
```
3. 加载遥感影像并进行预处理:
```python
image_path = 'path_to_remote_sensing_image'
remote_sensing_image = Image.open(image_path)
remote_sensing_image = remote_sensing_image.resize((512, 512)) # 按需调整图像尺寸
remote_sensing_image_array = image.img_to_array(remote_sensing_image)
remote_sensing_image_array = preprocess_input(remote_sensing_image_array)
remote_sensing_image_array = np.expand_dims(remote_sensing_image_array, axis=0)
```
4. 利用模型进行建筑物的自动提取:
```python
mask = model.predict(remote_sensing_image_array)
predicted_mask = np.argmax(mask, axis=-1)
predicted_mask = np.squeeze(predicted_mask)
```
5. 可选:将预测的掩码转换为二进制图像,便于可视化或后续分析:
```python
building_mask = np.zeros_like(predicted_mask)
building_mask[predicted_mask == 2] = 255
building_mask = Image.fromarray(building_mask.astype('uint8'))
```
这样,我们就完成了使用deeplabv3网络实现遥感影像中建筑物的自动提取。您可以根据具体的需求对代码进行进一步的优化和调整。
### 回答3:
使用DeepLabv3网络实现遥感影像中建筑物的自动提取可以分为以下几个步骤:
1. 数据准备:获取并准备遥感影像的训练集和测试集,需要包含有标注的建筑物区域。训练集应当包括影像及其对应的建筑物掩码。
2. 搭建DeepLabv3网络:使用Python及深度学习框架(如TensorFlow或PyTorch)搭建DeepLabv3网络。DeepLabv3是一个基于卷积神经网络的语义分割模型,可以实现像素级别的分类。通过将影像输入网络,可以得到每个像素点属于建筑物的概率。
3. 训练网络:使用训练集对DeepLabv3网络进行训练。训练过程中,可以使用交叉熵损失函数等评估网络输出的正确性,并使用反向传播算法更新网络参数,使网络逐渐优化。
4. 测试网络:使用测试集对训练好的DeepLabv3网络进行测试。将测试集中的影像输入网络,得到像素级别的建筑物预测结果。可以使用评价指标(如准确率、召回率、F1-Score等)来评估模型的性能。
5. 后处理:对网络输出的建筑物预测结果进行后处理,例如通过阈值化将概率转化为二值(建筑物/非建筑物),去除噪声等。
6. 应用模型:将训练好的DeepLabv3模型应用到新的遥感影像中,即可实现对该影像中建筑物的自动提取。
总结起来,使用DeepLabv3网络实现遥感影像中建筑物的自动提取需要进行数据准备、网络搭建、训练、测试及后处理等步骤。这个过程中,需要注意数据的准备和标注质量,网络的选择和配置,以及评估模型性能的指标选择。
阅读全文
相关推荐
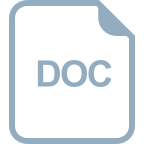
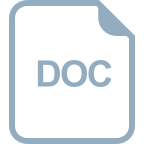
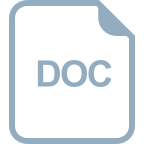
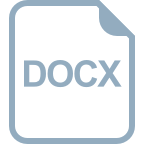
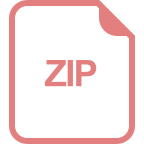
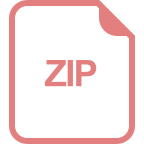
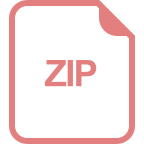
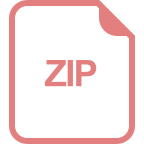
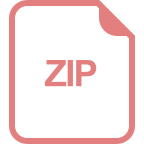
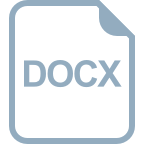
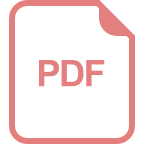
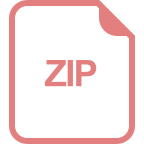
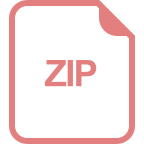
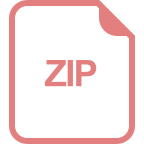
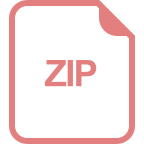
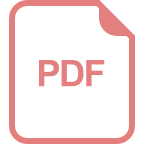
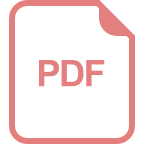
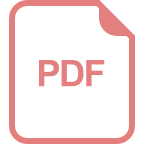
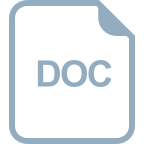