怎样用指针实现复制空串
时间: 2023-07-20 11:38:03 浏览: 115
可以使用以下代码来用指针实现复制空串:
```c
char* copy_str(char* str) {
char* copy = (char*)malloc(sizeof(char)); // 分配一个字符的空间
*copy = '\0'; // 将字符串的第一个字符设置为 null,表示空串
return copy;
}
int main() {
char* str = copy_str(""); // 复制空串
printf("%s", str); // 输出空串
free(str); // 释放内存
return 0;
}
```
解释一下代码:在 `copy_str` 函数中,我们先使用 `malloc` 分配一个字符的空间,然后将该字符设置为 null,表示空串。最后将指向该字符的指针返回。在 `main` 函数中,我们调用 `copy_str` 函数来复制空串,并将其输出。最后记得释放内存。
相关问题
小明学习了面向对象程序设计后,以字符指针作为数据成员设计了一个字符串类STRING,请你帮他实现输入输出运算符的重载(>>、<<)。 要求: (1)输入时以输入Enter键作为结束标志,允许输入长度不超过80个字符的可以包含空格、可以为空串的字符序列。 (2)输出时,仅输出字符串中的有效字符。字符串输出结束后,不输出换行符。
好的,我可以为你解答这个问题。
首先,我们需要在`STRING`类中声明友元函数`operator>>`和`operator<<`,用于重载输入输出运算符。代码如下:
```c++
class STRING
{
public:
// 构造函数
STRING();
// 重载输入输出运算符
friend istream& operator>>(istream& in, STRING& str);
friend ostream& operator<<(ostream& out, const STRING& str);
private:
char* data; // 字符串数据
int len; // 字符串长度
};
```
其中,`operator>>`是输入运算符的重载函数,参数为输入流对象和一个`STRING`对象的引用;`operator<<`是输出运算符的重载函数,参数为输出流对象和一个常量的`STRING`对象的引用。
接下来,我们可以在类外实现输入输出运算符的重载函数。代码如下:
```c++
istream& operator>>(istream& in, STRING& str)
{
char* buffer = new char[81]; // 定义一个缓冲区,用于存储输入的字符序列
in.getline(buffer, 81); // 从输入流中读取字符序列
int len = strlen(buffer); // 计算字符序列的长度
if (len > 0) // 如果字符序列非空
{
str.data = new char[len + 1]; // 分配足够的内存空间
strncpy(str.data, buffer, len); // 复制字符序列到字符串对象中
str.data[len] = '\0'; // 在字符串结尾添加一个空字符
str.len = len; // 更新字符串长度
}
else // 如果字符序列为空
{
str.data = new char[1]; // 分配一个字符的内存空间
str.data[0] = '\0'; // 添加一个空字符
str.len = 0; // 更新字符串长度
}
delete[] buffer; // 释放缓冲区内存空间
return in;
}
ostream& operator<<(ostream& out, const STRING& str)
{
for (int i = 0; i < str.len; i++)
{
out << str.data[i]; // 逐个输出字符串中的字符
}
return out;
}
```
在`operator>>`中,我们定义了一个缓冲区`buffer`,用于存储输入的字符序列。然后,我们使用`getline`函数从输入流对象中读取字符序列,最大长度为80个字符,以Enter键作为结束标志。接着,我们计算字符序列的长度,如果长度大于0,则分配足够的内存空间,并将字符序列复制到字符串对象中;如果长度为0,则分配一个字符的内存空间,并添加一个空字符。最后,我们释放缓冲区内存空间,并返回输入流对象的引用。
在`operator<<`中,我们逐个输出字符串中的有效字符,即前`len`个字符,然后返回输出流对象的引用。
通过以上的重载操作,我们就可以在程序中方便地使用输入输出运算符来操作`STRING`类对象了。
编写“串块链存储表示头文件.h”,其中包含:初始化空串、生成一个其值等于串常量的串、复制串、判空串、串比较、串联接、取子串、求串长、串的模式匹配、显示串等基本操作。 2编写“串块链主程序.cpp”,调用以上函数。
在C++中,我们可以创建一个名为`StringLinkList.h`的头文件来定义一个简单的字符串链表结构和相关的功能。这里是一个简化的实现:
```cpp
// StringLinkList.h
#ifndef STRINGLINKLIST_H
#define STRINGLINKLIST_H
#include <iostream>
#include <string>
class Node {
public:
std::string data;
Node* next;
// 构造函数和析构函数
Node(const std::string& data = "") : data(data), next(nullptr) {}
~Node() {}
};
class StringLinkList {
private:
Node* head;
public:
// 初始化空链表
StringLinkList() : head(nullptr) {}
// 初始化与给定字符串同值的链表
void initialize(const std::string& value);
// 复制当前链表
void copyFrom(StringLinkList& src);
// 判空
bool isEmpty() const;
// 比较两个链表是否相等
bool equals(StringLinkList& other) const;
// 串联接
void concatenate(const std::string& str);
// 取子串
std::string substr(int start, int length = -1) const;
// 求串长
int length() const;
// 模式匹配
bool isSubstringPresent(const std::string& pattern) const;
// 显示链表
void display() const;
};
#endif // STRINGLINKLIST_H
```
接下来,我们会为这个头文件编写对应的实现部分(`StringLinkList.cpp`),包括各个函数的细节:
```cpp
// StringLinkList.cpp
#include "StringLinkList.h"
// 实现函数
void StringLinkList::initialize(const std::string& value) {
if (!value.empty()) {
head = new Node(value);
}
}
void StringLinkList::copyFrom(StringLinkList& src) {
if (src.head != nullptr) {
Node* newNode = new Node(src.head->data);
head = newNode;
Node* temp = head;
while (src.head != nullptr) {
temp->next = new Node(src.head->data);
temp = temp->next;
src.head = src.head->next;
}
temp->next = nullptr;
}
}
bool StringLinkList::isEmpty() const {
return head == nullptr;
}
... // 其他函数的实现...
```
在这个例子中,我们创建了一个名为`StringLinkList`的类,它包含一个链表节点的指针`head`以及一些基本操作。`StringLinkList.cpp`中包含了所有这些函数的具体实现。
至于`main.cpp`文件,你可以按照以下方式调用这些函数:
```cpp
#include "StringLinkList.h"
#include <iostream>
int main() {
StringLinkList list1;
list1.initialize("Hello, World!");
// 使用其他函数操作list1...
StringLinkList list2;
list2.copyFrom(list1); // 复制list1的内容到list2
... // 更多操作
return 0;
}
```
阅读全文
相关推荐
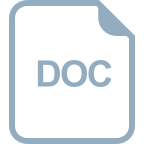
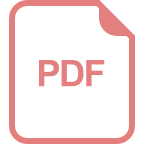
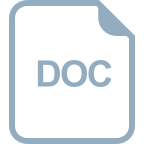
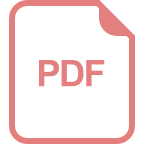
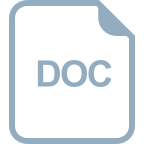
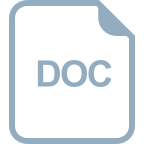
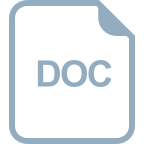
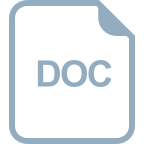
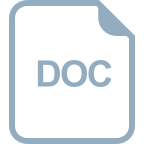
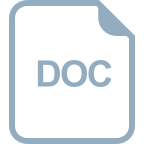
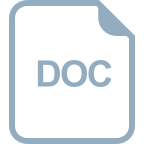




