hook、 hoc、 render props举个例子
时间: 2023-07-10 19:03:55 浏览: 169
好的,以下是一个简单的示例:
假设有一个组件 `Counter`,它可以接受一个 `count` 属性用于控制计数器的初始值,同时提供一个 `increment` 方法用于增加计数器的值。我们来分别使用 `hook`、`hoc`、`render props` 来实现这个组件。
1. 使用 `hook`
使用 `useState` hook 来管理计数器的状态,并通过 `useCallback` hook 来创建 `increment` 方法,代码如下:
```
import React, { useState, useCallback } from 'react';
function Counter({ count }) {
const [value, setValue] = useState(count);
const increment = useCallback(() => {
setValue(value => value + 1);
}, []);
return (
<div>
<p>{value}</p>
<button onClick={increment}>+1</button>
</div>
);
}
```
2. 使用 `hoc`
使用高阶组件(Higher-Order Component,即 `hoc`)来将 `count` 属性和 `increment` 方法注入到 `Counter` 组件中,代码如下:
```
import React from 'react';
function withCounter(WrappedComponent) {
return class extends React.Component {
state = {
value: this.props.count || 0
}
increment = () => {
this.setState(prevState => ({ value: prevState.value + 1 }));
}
render() {
return (
<WrappedComponent
value={this.state.value}
increment={this.increment}
{...this.props}
/>
);
}
}
}
function Counter({ value, increment }) {
return (
<div>
<p>{value}</p>
<button onClick={increment}>+1</button>
</div>
);
}
export default withCounter(Counter);
```
3. 使用 `render props`
通过将一个函数作为 `Counter` 组件的子组件,并将 `count` 属性和 `increment` 方法作为该函数的参数传递,来实现 `render props`。代码如下:
```
import React, { useState, useCallback } from 'react';
function Counter({ children, count }) {
const [value, setValue] = useState(count);
const increment = useCallback(() => {
setValue(value => value + 1);
}, []);
return children(value, increment);
}
function App() {
return (
<Counter count={0}>
{(value, increment) => (
<div>
<p>{value}</p>
<button onClick={increment}>+1</button>
</div>
)}
</Counter>
);
}
export default App;
```
阅读全文
相关推荐
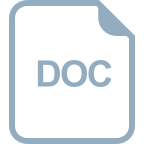
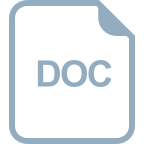
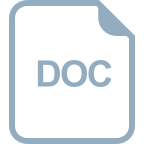
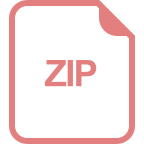
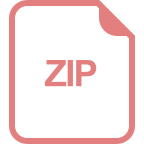
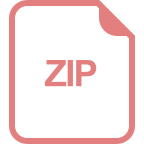
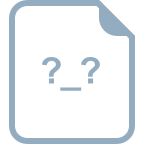
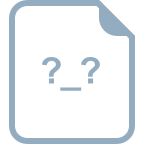
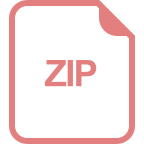
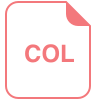
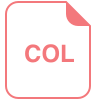
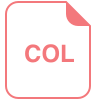
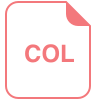
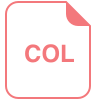
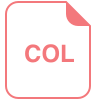

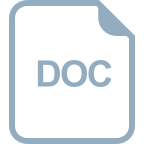
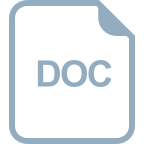
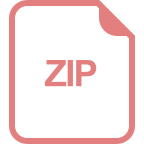