通过at指令at+bmcmd=upgrade指令实现升级,请具体阐述一下设备通过url下载升级包的恶流程,和建立连接,下载升级包,校验的具体实现的c语言代码
时间: 2023-12-06 10:02:31 浏览: 31
设备通过url下载升级包的流程可以分为以下几步:
1. 建立TCP连接
设备通过at+bmcmd=upgrade指令连上服务器,然后通过AT命令发送建立TCP连接请求,例如:
```
AT+QIOPEN="TCP","example.com",80
```
其中,example.com为升级包所在的服务器地址,80为服务器的端口号。
2. 发送HTTP GET请求获取升级包
建立TCP连接之后,设备需要发送HTTP GET请求获取升级包。具体代码如下:
```
AT+QISEND
GET /upgrade.bin HTTP/1.1\r\n
Host: example.com\r\n
Connection: keep-alive\r\n\r\n
AT+QIRD=0,1024
```
其中,/upgrade.bin为升级包的URL地址。
3. 接收升级包并校验
设备通过AT命令接收升级包,并对升级包进行校验。具体代码如下:
```
AT+QIRD=0,1024
```
以上代码表示从TCP连接中读取1024字节的数据。读取到的数据需要进行校验,确保接收到的是正确的升级包。
完整的C语言代码如下:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define APN "cmnet" // APN名称
#define USER "" // 用户名
#define PWD "" // 密码
#define SERVER_IP "example.com" // 服务器IP地址
#define SERVER_PORT 80 // 服务器端口号
void at_send(const char *at_cmd) {
printf("AT command: %s\n", at_cmd);
// 发送AT命令
}
void at_recv(char *buf, int len) {
// 从串口读取AT命令回复
}
int tcp_connect() {
// 建立TCP连接
char at_cmd[100];
snprintf(at_cmd, sizeof(at_cmd), "AT+QICSGP=1,\"%s\",\"%s\",\"%s\"", APN, USER, PWD);
at_send(at_cmd); // 设置APN信息
at_send("AT+QIACT=1"); // 激活PDP上下文
snprintf(at_cmd, sizeof(at_cmd), "AT+QIOPEN=\"TCP\",\"%s\",%d", SERVER_IP, SERVER_PORT);
at_send(at_cmd); // 建立TCP连接
char buf[1024];
at_recv(buf, sizeof(buf)); // 读取AT命令回复
if (strstr(buf, "+QIOPEN: 0,0") == NULL) {
// 连接失败
return -1;
}
return 0;
}
void tcp_disconnect() {
// 断开TCP连接
at_send("AT+QICLOSE");
at_send("AT+QIDEACT=1");
}
int http_get(const char *url, char *buf, int len) {
// 发送HTTP GET请求
char at_cmd[200];
snprintf(at_cmd, sizeof(at_cmd), "AT+QHTTPURL=%d,30", strlen(url));
at_send(at_cmd);
at_send(url);
char recv_buf[1024];
at_recv(recv_buf, sizeof(recv_buf));
if (strstr(recv_buf, "CONNECT") == NULL) {
// 请求失败
return -1;
}
at_send("AT+QHTTPGET=80");
at_recv(recv_buf, sizeof(recv_buf));
if (strstr(recv_buf, "+QHTTPGET: 0") == NULL) {
// 请求失败
return -1;
}
int total_len = 0;
while (1) {
at_send("AT+QHTTPREAD=80");
at_recv(recv_buf, sizeof(recv_buf));
char *data_ptr = strstr(recv_buf, "+QHTTPREAD: ");
if (data_ptr == NULL) {
// 读取数据失败
return -1;
}
data_ptr += strlen("+QHTTPREAD: ");
int data_len = strtol(data_ptr, NULL, 10);
if (data_len == 0) {
// 数据读取完成
break;
}
if (total_len + data_len > len) {
// 数据超过缓存区大小,直接退出
return -1;
}
at_recv(buf + total_len, data_len);
total_len += data_len;
}
return total_len;
}
int main() {
if (tcp_connect() != 0) {
printf("TCP connect failed\n");
return -1;
}
char buf[1024];
if (http_get("/upgrade.bin", buf, sizeof(buf)) < 0) {
printf("HTTP GET failed\n");
tcp_disconnect();
return -1;
}
// 对升级包进行校验...
tcp_disconnect();
return 0;
}
```
相关推荐
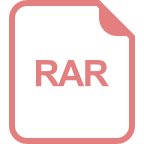
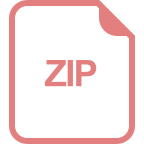














