poi-ooxml 读取excel中的date 变成字符串
时间: 2023-12-14 16:35:53 浏览: 242
读取 Excel 中的日期,可以使用 POI 的 DataFormatter 类将其转换为字符串。下面是一个示例代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.DataFormatter;
import org.apache.poi.ss.usermodel.DateUtil;
import org.apache.poi.xssf.usermodel.XSSFCell;
import org.apache.poi.xssf.usermodel.XSSFRow;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ReadExcel {
public static void main(String[] args) {
try {
FileInputStream file = new FileInputStream(new File("sample.xlsx"));
XSSFWorkbook workbook = new XSSFWorkbook(file);
XSSFSheet sheet = workbook.getSheetAt(0);
// Create a DataFormatter object to format the cell values
DataFormatter formatter = new DataFormatter();
// Loop through each row and column
for (int i = 0; i <= sheet.getLastRowNum(); i++) {
XSSFRow row = sheet.getRow(i);
if (row != null) {
for (int j = 0; j < row.getLastCellNum(); j++) {
XSSFCell cell = row.getCell(j);
if (cell != null) {
String cellValue = formatter.formatCellValue(cell);
if (DateUtil.isCellDateFormatted(cell)) {
Date date = cell.getDateCellValue();
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
cellValue = dateFormat.format(date);
}
System.out.print(cellValue + "\t");
} else {
System.out.print("\t");
}
}
System.out.println();
}
}
workbook.close();
file.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,我们首先读取 Excel 文件并获取第一个工作表。然后,我们使用 DataFormatter 类来格式化单元格的值。如果单元格包含日期值,则我们将其转换为字符串。最后,我们输出每个单元格的值。
注意:上面的示例代码假设日期格式为 "yyyy-MM-dd",您可以根据需要更改日期格式。
阅读全文
相关推荐
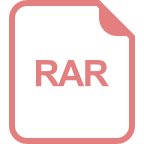
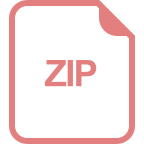
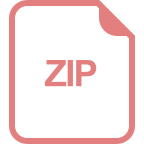
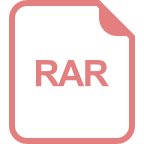
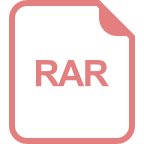
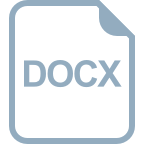
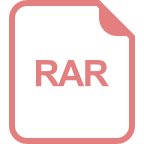
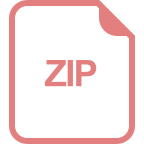
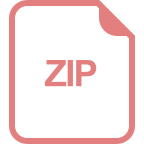
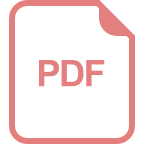
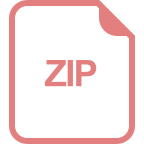
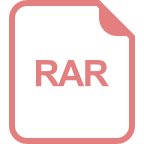
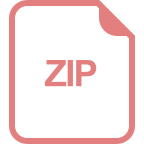
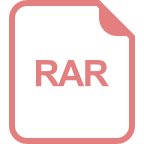
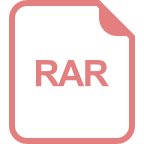
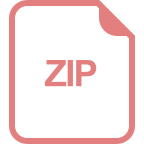
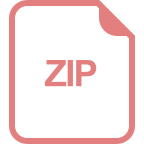