使用c语言编写一、设计游戏:五子棋; 要求:1、显示11*11的棋盘界面; (25分〉 2、两位选手能按照行棋规则落子(分别为黑白色); (25分) 3、能够判断棋局的胜负情况,并显示对弈结果; (25分) 4、背景音乐和落子音效,且能提供设置界面开启和关闭该功能;(20分>5、其他功能。
时间: 2024-03-11 13:43:34 浏览: 75
以下是一个使用 C 语言编写的五子棋游戏示例代码,基于控制台输出实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <Windows.h>
#include <conio.h>
#define BOARD_SIZE 11
#define BLACK 'X'
#define WHITE 'O'
char board[BOARD_SIZE][BOARD_SIZE];
void init_board() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
board[i][j] = '+';
}
}
}
void print_board() {
system("cls");
printf(" ");
for (int i = 0; i < BOARD_SIZE; i++) {
printf("%2d ", i);
}
printf("\n");
for (int i = 0; i < BOARD_SIZE; i++) {
printf("%4d ", i);
for (int j = 0; j < BOARD_SIZE; j++) {
printf("%2c ", board[i][j]);
}
printf("\n");
}
}
bool check_win(char player) {
// 检查行
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE - 4; j++) {
if (board[i][j] == player && board[i][j+1] == player && board[i][j+2] == player && board[i][j+3] == player && board[i][j+4] == player) {
return true;
}
}
}
// 检查列
for (int i = 0; i < BOARD_SIZE - 4; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (board[i][j] == player && board[i+1][j] == player && board[i+2][j] == player && board[i+3][j] == player && board[i+4][j] == player) {
return true;
}
}
}
// 检查正斜线
for (int i = 0; i < BOARD_SIZE - 4; i++) {
for (int j = 0; j < BOARD_SIZE - 4; j++) {
if (board[i][j] == player && board[i+1][j+1] == player && board[i+2][j+2] == player && board[i+3][j+3] == player && board[i+4][j+4] == player) {
return true;
}
}
}
// 检查反斜线
for (int i = 0; i < BOARD_SIZE - 4; i++) {
for (int j = 4; j < BOARD_SIZE; j++) {
if (board[i][j] == player && board[i+1][j-1] == player && board[i+2][j-2] == player && board[i+3][j-3] == player && board[i+4][j-4] == player) {
return true;
}
}
}
return false;
}
bool place_piece(char player, int row, int col) {
if (board[row][col] != '+') {
return false;
}
board[row][col] = player;
return true;
}
void play_game() {
int row, col;
char player = BLACK;
bool game_over = false;
while (!game_over) {
print_board();
printf("Player %c's turn. Please enter row and column: ", player);
scanf("%d %d", &row, &col);
if (row < 0 || row >= BOARD_SIZE || col < 0 || col >= BOARD_SIZE) {
printf("Invalid row or column. Please try again.\n");
continue;
}
if (!place_piece(player, row, col)) {
printf("The position is already occupied. Please try again.\n");
continue;
}
if (check_win(player)) {
printf("Player %c wins!\n", player);
game_over = true;
}
player = player == BLACK ? WHITE : BLACK;
}
}
int main() {
init_board();
play_game();
return 0;
}
```
该代码实现了棋盘的初始化、输出、棋子的落子和胜利判断等功能。玩家可以通过控制台输入行和列来落子,当有一方连成五子时,游戏结束并显示胜利者。
阅读全文
相关推荐
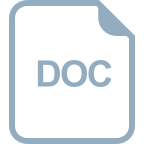
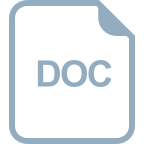
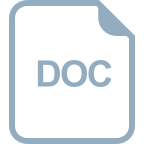

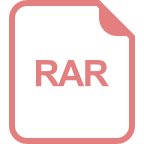
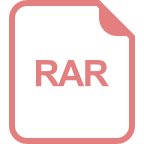
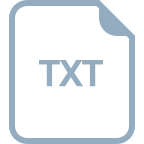
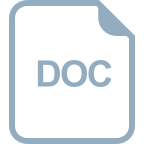
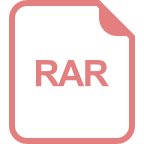
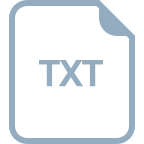
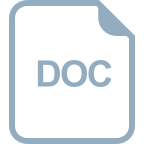
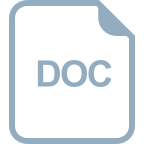
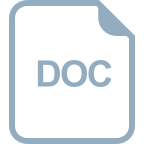
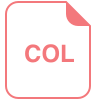
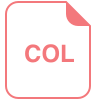
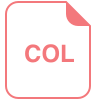

