基于Java写一个死锁避免银行家算法
时间: 2023-06-28 13:10:15 浏览: 91
银行家算法是一种资源分配和避免死锁的经典算法,下面是基于Java实现的示例代码:
```java
public class BankerAlgorithm {
private int[][] need; // 进程还需要的资源
private int[][] allocation; // 进程已经拥有的资源
private int[] available; // 系统剩余的资源
private int[][] max; // 进程最大需求资源
private int[] work; // 可用资源
private int processNum; // 进程数量
private int resourceNum; // 资源种类数量
private boolean[] finish; // 进程是否运行完成
public BankerAlgorithm(int[][] need, int[][] allocation, int[] available, int[][] max) {
this.need = need;
this.allocation = allocation;
this.available = available;
this.max = max;
this.processNum = need.length;
this.resourceNum = available.length;
this.work = Arrays.copyOf(available, resourceNum);
this.finish = new boolean[processNum];
}
/**
* 检查当前状态是否安全
*/
public boolean isSafe() {
int count = 0;
int[] safeSequence = new int[processNum];
while (count < processNum) {
boolean flag = false;
for (int i = 0; i < processNum; i++) {
if (!finish[i]) {
int j;
for (j = 0; j < resourceNum; j++) {
if (need[i][j] > work[j]) {
break;
}
}
if (j == resourceNum) {
for (int k = 0; k < resourceNum; k++) {
work[k] += allocation[i][k];
}
safeSequence[count++] = i;
finish[i] = true;
flag = true;
}
}
}
if (!flag) {
return false;
}
}
System.out.print("Safe sequence: ");
for (int i = 0; i < processNum; i++) {
System.out.print(safeSequence[i] + " ");
}
System.out.println();
return true;
}
/**
* 分配资源给进程
*/
public boolean allocate(int processId, int[] request) {
for (int i = 0; i < resourceNum; i++) {
if (request[i] > need[processId][i] || request[i] > available[i]) {
return false;
}
}
for (int i = 0; i < resourceNum; i++) {
available[i] -= request[i];
allocation[processId][i] += request[i];
need[processId][i] -= request[i];
}
return true;
}
/**
* 释放已分配资源
*/
public boolean release(int processId, int[] release) {
for (int i = 0; i < resourceNum; i++) {
if (release[i] > allocation[processId][i]) {
return false;
}
}
for (int i = 0; i < resourceNum; i++) {
available[i] += release[i];
allocation[processId][i] -= release[i];
need[processId][i] += release[i];
}
return true;
}
}
```
BankerAlgorithm类中包含了资源分配和避免死锁的方法,其中isSafe()方法可以检查当前状态是否安全,allocate()方法可以分配资源给进程,release()方法可以释放已分配资源。可以根据具体的业务场景进行调用。
阅读全文
相关推荐
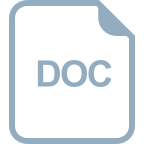
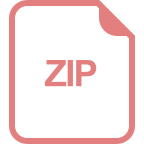
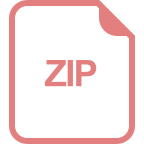
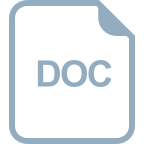
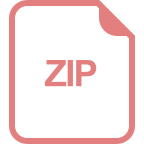
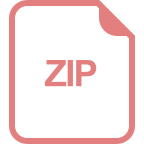
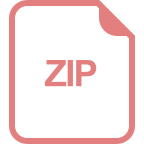
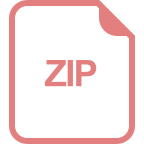
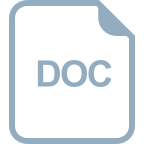
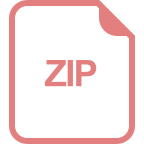
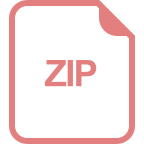
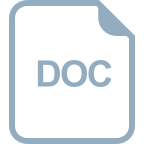
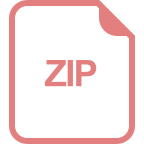
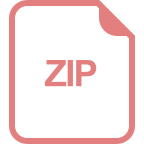
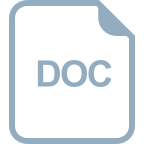
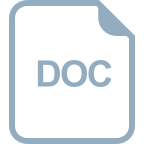
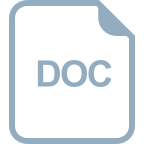