生成文件导入导出的前端页面代码,可以使用不同请求方式并且携带token
时间: 2024-03-10 11:43:49 浏览: 225
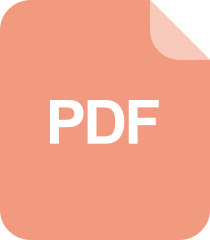
vue 导出文件,携带请求头token操作
生成文件导入导出的前端页面代码可以使用以下代码片段作为参考:
```javascript
// 导入文件请求
const importFile = (file, token) => {
return axios.post('/api/import', file, {
headers: {
'Authorization': `Bearer ${token}`,
'Content-Type': 'multipart/form-data'
}
})
}
// 导出文件请求
const exportFile = (params, token) => {
return axios.get('/api/export', {
params: params,
headers: {
'Authorization': `Bearer ${token}`
},
responseType: 'blob'
})
}
// 文件上传组件
<template>
<div>
<input type="file" ref="fileInput" @change="importFile">
</div>
</template>
<script>
import axios from 'axios'
export default {
methods: {
importFile() {
const file = this.$refs.fileInput.files[0]
const token = 'your-token-here'
importFile(file, token)
.then(response => {
// 处理导入结果
})
.catch(error => {
// 处理导入错误
})
}
}
}
</script>
// 文件导出组件
<template>
<div>
<button @click="exportFile">导出文件</button>
</div>
</template>
<script>
import axios from 'axios'
export default {
methods: {
exportFile() {
const params = {
// 导出文件的参数
}
const token = 'your-token-here'
exportFile(params, token)
.then(response => {
const blob = new Blob([response.data])
const filename = `${params.filename}.${params.format}`
const url = window.URL.createObjectURL(blob)
const link = document.createElement('a')
link.href = url
link.download = filename
link.click()
window.URL.revokeObjectURL(url)
})
.catch(error => {
// 处理导出错误
})
}
}
}
</script>
```
在这里,我们使用了 Axios 库来发送导入和导出文件的请求。在导入请求中,我们使用了 `multipart/form-data` 类型的请求头,并将 token 放在了 `Authorization` 请求头中。在导出请求中,我们使用了 `blob` 类型的响应类型,并且同样将 token 放在了 `Authorization` 请求头中。
在前端页面中,我们分别创建了一个文件上传组件和一个文件导出组件。在上传组件中,我们监听了文件选择框的 `change` 事件,并在选择文件后调用了 `importFile` 方法来发送导入请求。在导出组件中,我们创建了一个按钮,并在点击按钮时调用了 `exportFile` 方法来发送导出请求。在导出请求的成功回调中,我们使用了 `Blob` 和 `URL.createObjectURL` 方法来创建一个下载链接,并自动下载导出的文件。
阅读全文
相关推荐
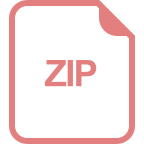
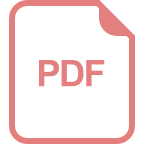
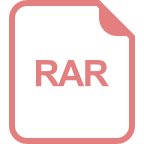
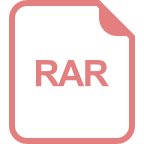
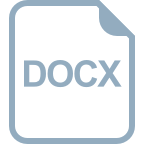
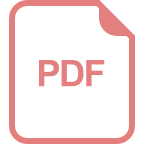
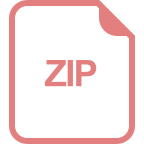
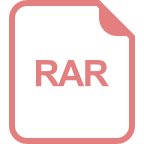
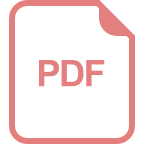
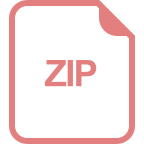
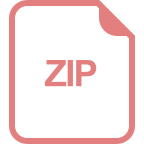
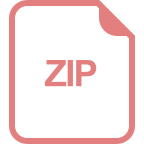