vue3中批量创建时间,要求例如开始时间为2023-5-10 结束时间为2023-6-10,要求每周2,每周3,每周4创建时间,每周几是一个变量,不固定,不要写死
时间: 2024-02-26 11:58:35 浏览: 13
您好,针对您的问题,我可以给出一个基于 Vue.js 3.x 的实现方案。
首先,我们需要将开始时间和结束时间转换为 JavaScript 中的 Date 对象,方便后续的计算。可以使用如下代码:
```javascript
const startDate = new Date('2023-05-10');
const endDate = new Date('2023-06-10');
```
然后,我们需要确定创建时间的日期,也就是每周几。假设这个变量是一个数组,存储了每周需要创建时间的日期,可以使用如下代码获取当前日期是每周几:
```javascript
const weekDays = [2, 3, 4]; // 需要创建时间的日期
const today = new Date().getDay(); // 获取当前日期是每周几,0 表示周日,1 表示周一,以此类推
const isWeekDay = weekDays.includes(today); // 判断当前日期是否需要创建时间
```
接下来,我们需要遍历从开始时间到结束时间的每一天,判断是否需要创建时间。如果需要,就将创建时间的信息存储到一个数组中。可以使用如下代码实现:
```javascript
const timeList = []; // 存储创建时间的数组
let currentDate = startDate; // 当前的日期,初始值为开始时间
while (currentDate <= endDate) {
const currentDay = currentDate.getDay();
if (weekDays.includes(currentDay)) {
// 如果当前日期是需要创建时间的日期,则将创建时间的信息存储到数组中
const time = {
date: currentDate.toLocaleDateString(), // 时间的日期,格式为 YYYY/M/D
time: '10:00', // 创建时间的具体时间,这里暂时写死为 10:00
};
timeList.push(time);
}
// 将当前日期加一天
currentDate.setDate(currentDate.getDate() + 1);
}
```
最后,我们可以在 Vue 的模板中使用 v-for 指令渲染创建时间的列表。可以使用如下代码实现:
```html
<template>
<div>
<ul>
<li v-for="time in timeList" :key="time.date">
{{ time.date }} {{ time.time }}
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
weekDays: [2, 3, 4],
startDate: new Date('2023-05-10'),
endDate: new Date('2023-06-10'),
timeList: [],
};
},
mounted() {
let currentDate = this.startDate;
while (currentDate <= this.endDate) {
const currentDay = currentDate.getDay();
if (this.weekDays.includes(currentDay)) {
const time = {
date: currentDate.toLocaleDateString(),
time: '10:00',
};
this.timeList.push(time);
}
currentDate.setDate(currentDate.getDate() + 1);
}
},
};
</script>
```
这样,就可以实现批量创建时间的功能了。需要注意的是,这里的创建时间的具体时间是写死的,如果需要根据实际需求进行调整。另外,由于涉及到日期的比较和计算,可能存在时区的影响,需要注意时区的设置。
相关推荐
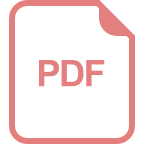
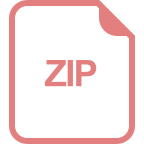
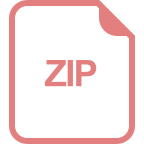














