用C语言写链表的各个操作
时间: 2023-12-29 17:51:25 浏览: 38
链表是一种常见的数据结构,它可以用来存储和管理大量的数据。下面是用C语言写链表的各个操作:
1. 定义节点结构体
```c
struct Node {
int data;
struct Node* next;
};
```
2. 创建链表
```c
struct Node* createList(int n) {
struct Node* head = NULL;
struct Node* tail = NULL;
for (int i = 0; i < n; i++) {
int data;
printf("请输入第%d个节点的数据:", i + 1);
scanf("%d", &data);
struct Node* node = (struct Node*)malloc(sizeof(struct Node));
node->data = data;
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
```
3. 插入节点
```c
struct Node* insertNode(struct Node* head, int index, int data) {
if (index < 0) {
printf("插入位置不合法\n");
return head;
}
struct Node* node = (struct Node*)malloc(sizeof(struct Node));
node->data = data;
node->next = NULL;
if (index == 0) {
node->next = head;
head = node;
} else {
struct Node* p = head;
int i = 0;
while (p != NULL && i < index - 1) {
p = p->next;
i++;
}
if (p == NULL) {
printf("插入位置不合法\n");
return head;
}
node->next = p->next;
p->next = node;
}
return head;
}
```
4. 删除节点
```c
struct Node* deleteNode(struct Node* head, int index) {
if (index < 0) {
printf("删除位置不合法\n");
return head;
}
if (index == 0) {
struct Node* p = head;
head = head->next;
free(p);
} else {
struct Node* p = head;
int i = 0;
while (p != NULL && i < index - 1) {
p = p->next;
i++;
}
if (p == NULL || p->next == NULL) {
printf("删除位置不合法\n");
return head;
}
struct Node* q = p->next;
p->next = q->next;
free(q);
}
return head;
}
```
5. 查找节点
```c
int findNode(struct Node* head, int data) {
struct Node* p = head;
int i = 0;
while (p != NULL) {
if (p->data == data) {
return i;
}
p = p->next;
i++;
}
return -1;
}
```
6. 遍历链表
```c
void traverseList(struct Node* head) {
struct Node* p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
```
7. 销毁链表
```c
void destroyList(struct Node* head) {
struct Node* p = head;
while (p != NULL) {
struct Node* q = p;
p = p->next;
free(q);
}
}
```
相关推荐
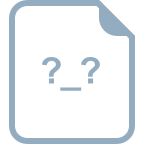














