python 移动窗口
时间: 2025-01-07 10:47:37 浏览: 3
### Python 中实现滑动窗口算法
#### 无重复字符的最长子串问题示例
对于“无重复字符的最长子串”的问题,可以通过维护一个字典来记录字符最近一次出现的位置,并利用两个指针表示当前窗口的起始位置和结束位置。当遇到已经存在于字典中的字符时,则更新左边界以排除掉旧版本的存在[^1]。
```python
def length_of_longest_substring(s: str) -> int:
char_map = {}
left = 0
max_length = 0
for right in range(len(s)):
if s[right] in char_map:
left = max(char_map[s[right]] + 1, left)
char_map[s[right]] = right
max_length = max(max_length, right - left + 1)
return max_length
```
此函数接收字符串 `s` 并返回其不含重复字符的最长子串长度。
#### 计算固定大小窗口内的最大和
另一个常见的应用场景是在给定数组中找到指定宽度k的连续子数组的最大总和。这里定义了一个名为 `max_sum_sliding_window` 的方法来完成这项工作[^2]:
```python
from collections import deque
def max_sum_sliding_window(nums, k):
n = len(nums)
if not nums or k <= 0:
return None
window = deque()
res = []
for i in range(n):
# 移除队列头部不在当前窗口范围内的索引
while window and window[0] < i - k + 1:
window.popleft()
# 维护单调递减队列
while window and nums[i] >= nums[window[-1]]:
window.pop()
window.append(i)
# 当遍历到第k个元素之后开始收集结果
if i >= k - 1:
res.append(nums[window[0]])
return sum(res)//len(res)
arr = [4, 2, 1, 7, 8, 1, 2, 8, 1, 0]
k = 3
result = max_sum_sliding_window(arr, k)
print("滑动窗口的最大和为:", result)
```
上述代码实现了求解特定区间内数值之和最大的功能,并打印最终的结果。
阅读全文
相关推荐












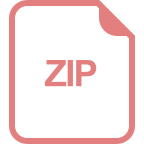



