Spring Security如何在接口中登录基于表单方式,后台接口完成登录
时间: 2024-05-11 14:18:15 浏览: 185
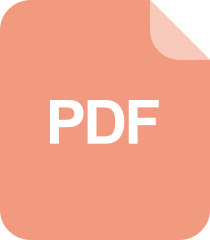
Spring Security 表单登录功能的实现方法
Spring Security提供了一些配置和类,可以让开发者轻松地实现表单登录认证和授权。下面是一个基于表单方式登录的Spring Security接口登录示例:
1. 配置Spring Security
在Spring Security的配置中,需要配置一个登录页面、登录请求处理URL、登录成功后的处理器、登录失败后的处理器等。
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests().anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.loginProcessingUrl("/doLogin")
.successHandler(new LoginSuccessHandler())
.failureHandler(new LoginFailureHandler())
.permitAll();
}
}
```
2. 实现登录接口
在登录请求处理URL上,我们需要实现一个接口,来处理用户提交的表单数据。在这个接口中,我们可以使用Spring Security提供的API来进行登录认证。
```java
@RestController
public class LoginController {
@PostMapping("/doLogin")
public String doLogin(HttpServletRequest request, HttpServletResponse response) {
String username = request.getParameter("username");
String password = request.getParameter("password");
UsernamePasswordAuthenticationToken token = new UsernamePasswordAuthenticationToken(username, password);
try {
Authentication authentication = authenticationManager.authenticate(token);
SecurityContextHolder.getContext().setAuthentication(authentication);
return "success";
} catch (AuthenticationException e) {
return "error";
}
}
}
```
在这个接口中,我们首先从请求中获取用户名和密码,然后创建一个UsernamePasswordAuthenticationToken对象,并使用authenticationManager.authenticate()方法来进行登录认证。如果认证成功,我们将用户信息保存在SecurityContextHolder中,返回"success"。如果认证失败,返回"error"。
3. 实现登录成功和失败的处理器
在登录成功或失败后,我们需要做出相应的处理。可以通过实现AuthenticationSuccessHandler和AuthenticationFailureHandler接口来实现登录成功和失败的处理器。
```java
public class LoginSuccessHandler implements AuthenticationSuccessHandler {
@Override
public void onAuthenticationSuccess(HttpServletRequest request, HttpServletResponse response, Authentication authentication) throws IOException, ServletException {
response.setContentType("application/json;charset=utf-8");
PrintWriter out = response.getWriter();
out.write("{\"status\":\"success\",\"msg\":\"登录成功\"}");
out.flush();
out.close();
}
}
public class LoginFailureHandler implements AuthenticationFailureHandler {
@Override
public void onAuthenticationFailure(HttpServletRequest request, HttpServletResponse response, AuthenticationException e) throws IOException, ServletException {
response.setContentType("application/json;charset=utf-8");
PrintWriter out = response.getWriter();
out.write("{\"status\":\"error\",\"msg\":\"登录失败\"}");
out.flush();
out.close();
}
}
```
在这两个处理器中,我们分别处理了登录成功和失败的情况,并返回相应的JSON数据。
这样,我们就完成了基于表单方式的Spring Security接口登录。
阅读全文
相关推荐
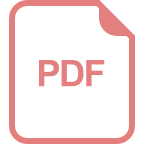
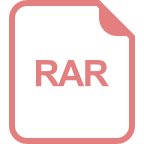

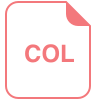
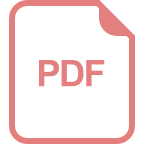

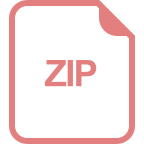
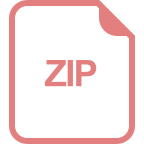
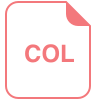

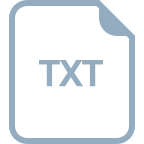
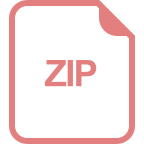
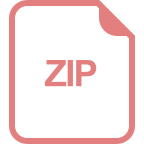

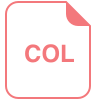
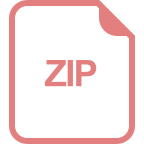
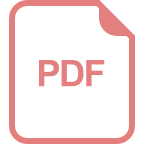
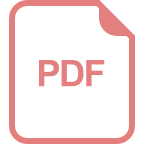