bagging python
时间: 2023-07-14 16:01:40 浏览: 61
Bagging (Bootstrap Aggregating) is a machine learning ensemble technique that combines multiple models to improve prediction accuracy. In Python, you can use the scikit-learn library to implement bagging.
To perform bagging in Python, you can follow these steps:
1. Import the necessary libraries:
```python
from sklearn.ensemble import BaggingClassifier
from sklearn.tree import DecisionTreeClassifier
```
2. Create an instance of the base model (e.g., Decision Tree):
```python
base_model = DecisionTreeClassifier()
```
3. Create an instance of the BaggingClassifier and specify the number of base models (n_estimators) and other parameters:
```python
bagging_model = BaggingClassifier(base_model, n_estimators=10, max_samples=0.5, max_features=0.5)
```
Here, `n_estimators` represents the number of base models to use, `max_samples` specifies the fraction of samples to draw from the training set for each base model, and `max_features` represents the fraction of features to consider for each base model.
4. Fit the bagging model to your training data:
```python
bagging_model.fit(X_train, y_train)
```
Here, `X_train` represents the training features and `y_train` represents the corresponding labels.
5. Use the bagging model to make predictions:
```python
y_pred = bagging_model.predict(X_test)
```
Here, `X_test` represents the test features, and `y_pred` will contain the predicted labels for the test data.
This is a basic example of how to implement bagging in Python using scikit-learn. Keep in mind that you can also use bagging with other types of models, not just decision trees.
相关推荐
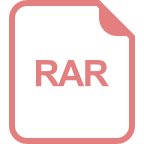











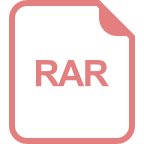
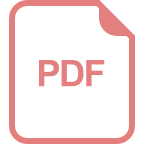
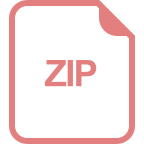
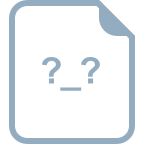