编写函数void myitoa(int n,char *str),其功能是将一个整数转换为对应的字符串。
时间: 2024-04-30 18:17:47 浏览: 15
好的,以下是函数的实现:
```c
#include <stdio.h>
#include <string.h>
void reverse(char *str, int len) {
int i = 0, j = len - 1;
while (i < j) {
char temp = str[i];
str[i] = str[j];
str[j] = temp;
i++;
j--;
}
}
void myitoa(int n, char *str) {
int i = 0;
if (n == 0) {
str[i++] = '0';
str[i] = '\0';
return;
}
int sign = (n < 0) ? -1 : 1;
n = (sign == -1) ? -n : n;
while (n) {
str[i++] = n % 10 + '0';
n = n / 10;
}
if (sign == -1) {
str[i++] = '-';
}
str[i] = '\0';
reverse(str, i);
}
int main() {
int n = 12345;
char str[100];
myitoa(n, str);
printf("The integer %d is represented as string %s\n", n, str);
return 0;
}
```
函数 `myitoa` 的参数为整数 `n` 和字符串指针 `str`,返回值为 `void`。函数中使用了一个辅助函数 `reverse`,它用于将字符串反转。
首先判断 `n` 是否为 0,如果是,直接将字符串赋值为 "0";否则,判断 `n` 是否为负数,如果是,将 `sign` 设为 -1,并将 `n` 取反。接下来,从低位到高位依次将 `n` 的每一位数字转换为字符,并存储到字符串中。如果 `n` 是负数,再在字符串的开头加上一个负号。最后,将字符串反转,使得字符串中的数字按照从高位到低位的顺序排列。
在本示例中,程序将整数 12345 转换为字符串 "12345"。
相关推荐
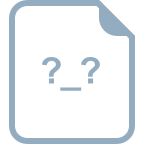
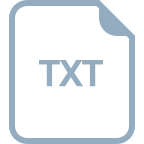
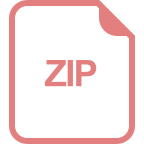
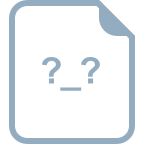
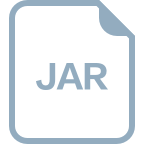
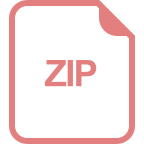
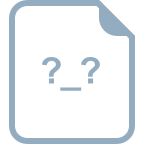
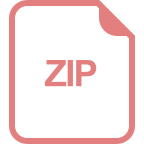
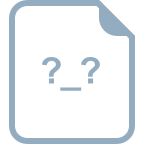