用Java编制一个读单词过程,从输入的源程序中,识别出各个具有独立意义的单词,即基本保留字、标识符、常数、运算符、分隔符五大类。并依次输出各个单词的内部编码及单词符号自身值。
时间: 2023-11-26 16:02:42 浏览: 59
以下是一个简单的Java程序,用于读取源代码并识别出各个单词:
```java
import java.io.*;
public class WordScanner {
public static void main(String[] args) {
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
String line;
while ((line = reader.readLine()) != null) {
String[] words = line.split("\\s+"); // 根据空格分割单词
for (String word : words) {
if (isKeyword(word)) { // 判断是否为关键字
System.out.println("Keyword: " + word);
} else if (isIdentifier(word)) { // 判断是否为标识符
System.out.println("Identifier: " + word);
} else if (isConstant(word)) { // 判断是否为常数
System.out.println("Constant: " + word);
} else if (isOperator(word)) { // 判断是否为运算符
System.out.println("Operator: " + word);
} else if (isSeparator(word)) { // 判断是否为分隔符
System.out.println("Separator: " + word);
}
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
// 判断是否为关键字
private static boolean isKeyword(String word) {
String[] keywords = {"if", "else", "while", "for", "switch", "case", "break", "default", "int", "float", "double", "char", "boolean", "void", "class", "public", "private", "protected", "static", "final", "abstract", "extends", "implements", "interface", "new", "return", "this", "super"};
for (String keyword : keywords) {
if (word.equals(keyword)) {
return true;
}
}
return false;
}
// 判断是否为标识符
private static boolean isIdentifier(String word) {
return word.matches("[a-zA-Z_][a-zA-Z0-9_]*");
}
// 判断是否为常数
private static boolean isConstant(String word) {
return word.matches("\\d+");
}
// 判断是否为运算符
private static boolean isOperator(String word) {
String[] operators = {"+", "-", "*", "/", "%", "=", "==", "!=", ">", "<", ">=", "<=", "&&", "||", "!", "&", "|", "^", ">>", "<<", ">>>", "~", "+=", "-=", "*=", "/=", "%=", "++", "--", "<=>", "::"};
for (String operator : operators) {
if (word.equals(operator)) {
return true;
}
}
return false;
}
// 判断是否为分隔符
private static boolean isSeparator(String word) {
String[] separators = {"(", ")", "{", "}", "[", "]", ";", ",", ".", ":", "?", "!"};
for (String separator : separators) {
if (word.equals(separator)) {
return true;
}
}
return false;
}
}
```
该程序使用 `BufferedReader` 读取输入的源代码,并使用 `split` 方法根据空格分割出单词。然后,对于每个单词,使用不同的方法判断其类别并输出相应的信息。例如,`isKeyword` 方法用于判断一个单词是否为关键字,其原理是将所有关键字存储在一个数组中并逐一比较。类似地,`isIdentifier` 方法用于判断标识符,`isConstant` 方法用于判断常数,`isOperator` 方法用于判断运算符,`isSeparator` 方法用于判断分隔符。
以上仅是一个简单的示例程序,实际的单词扫描器需要考虑更多的语言特性和复杂性,如注释、字符串等。
相关推荐
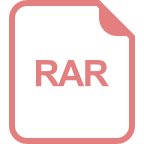
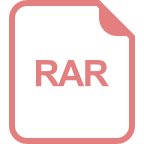
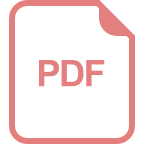












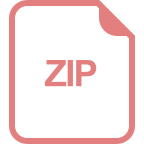
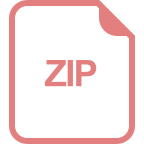