linux ncurses
时间: 2023-07-14 07:53:54 浏览: 108
ncurses是一个用于编写交互式终端界面的库,它提供了一些函数和数据结构,使得程序员可以在终端上方便地实现菜单、窗口、文本框等交互式界面元素。ncurses可以在多种Unix-like系统上使用,包括Linux。ncurses的功能包括:终端控制、窗口管理、颜色、键盘/鼠标输入、滚动、分页等等。通过使用ncurses库,程序员可以编写出非常复杂的交互式终端应用程序,比如图形化的文件管理器、文本编辑器等。
相关问题
Linux ncurses getch
The `getch()` function is a blocking input function in the ncurses library of Linux. It waits for the user to press a key on the keyboard and returns the corresponding integer value of the key pressed.
Here is a simple example of using `getch()` to read a single character from the user:
```
#include <ncurses.h>
int main()
{
initscr(); // initialize ncurses
cbreak(); // don't wait for the Enter key to be pressed
noecho(); // don't print the character on the screen
keypad(stdscr, TRUE); // enable function keys
printw("Press any key to continue...");
refresh(); // redraw the screen
getch(); // wait for a keypress
endwin(); // restore terminal settings
return 0;
}
```
In this example, `initscr()` initializes ncurses, `cbreak()` puts the terminal into cbreak mode (which means input is immediately available to the program without waiting for the Enter key to be pressed), `noecho()` disables the automatic printing of input characters to the screen, and `keypad()` enables function keys.
The `printw()` function prints a message to the screen, and `refresh()` redraws the screen. Finally, `getch()` waits for a keypress, and `endwin()` restores the terminal settings before exiting the program.
Note: The return value of `getch()` is an integer, but most keys on the keyboard have an ASCII code that corresponds to their value. For example, the ASCII code for the 'A' key is 65. You can use `getch()` to read the ASCII code of a key by casting the result to a `char`. For example:
```
char ch = (char)getch();
```
Linux ncurses getch() 检测F1键退出
以下是一个使用ncurses库的C++示例代码,用于检测用户是否按下F1键并退出程序:
```
#include <ncurses.h>
int main() {
initscr(); // 初始化屏幕
cbreak(); // 禁用缓冲
noecho(); // 禁用回显
keypad(stdscr, TRUE); // 启用功能键
int ch;
while ((ch = getch()) != KEY_F(1)) { // 持续读取输入
// 处理其他输入
}
endwin(); // 关闭屏幕
return 0;
}
```
在此示例中,`getch()`函数用于读取用户输入。如果用户按下F1键,则`KEY_F(1)`宏将返回真值,程序将退出。如果需要检测其他按键,可以使用ncurses库中的其他宏。
阅读全文
相关推荐













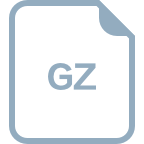