springboot redis session共享
时间: 2023-05-31 16:19:41 浏览: 164
### 回答1:
Spring Boot可以通过Redis实现Session共享,具体步骤如下:
1. 在pom.xml中添加Redis和Spring Session的依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.session</groupId>
<artifactId>spring-session-data-redis</artifactId>
</dependency>
```
2. 配置Redis连接信息:
```
spring.redis.host=127.0.0.1
spring.redis.port=6379
spring.redis.password=
```
3. 配置Spring Session:
```
@Configuration
@EnableRedisHttpSession
public class RedisSessionConfig {
@Bean
public LettuceConnectionFactory connectionFactory() {
return new LettuceConnectionFactory();
}
}
```
4. 在Controller中使用Session:
```
@GetMapping("/setSession")
public String setSession(HttpServletRequest request) {
request.getSession().setAttribute("name", "张三");
return "设置Session成功";
}
@GetMapping("/getSession")
public String getSession(HttpServletRequest request) {
String name = (String) request.getSession().getAttribute("name");
return "Session中的name值为:" + name;
}
```
这样就可以实现Session共享了。
### 回答2:
在前端开发中,用户会进行登录操作来访问网站的各种功能,而登录状态的维护和管理需要使用到session技术。session是在Web服务器端存储的一个会话状态,用于跨不同请求维护同一个用户的状态信息。但是,如果Web服务器存在负载均衡,session就会出现不一致的问题,这时可以使用Redis来实现session共享。
Redis是一种高性能的NoSQL数据库,支持快速地读写操作和高并发处理。Redis可以将session存储在内存中,提供了集群化的分布式存储方案,能够实现session共享。在SpringBoot中,我们可以使用Spring Session框架来进行Redis session共享的配置。
首先,在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.session</groupId>
<artifactId>spring-session-data-redis</artifactId>
</dependency>
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
</dependency>
```
然后,需要在application.properties文件中添加以下配置信息:
```
#Redis配置
spring.redis.host = localhost
spring.redis.port = 6379
spring.redis.password =
spring.redis.database = 0
#Session配置
spring.session.store-type = redis
```
其中,spring.redis.host和spring.redis.port是连接Redis的地址和端口号,如果需要密码进行连接,可以在spring.redis.password中设置;spring.redis.database表示在Redis中的数据库索引,默认为0。最后,spring.session.store-type设置为redis,表示使用Redis存储session信息。
最后,我们需要在代码中使用session来完成操作。
在控制器中,可以使用@SessionAttributes注解来保存session中的数据,如下所示:
```
@Controller
@SessionAttributes({"username", "email"})
public class UserController {
//...
}
```
在访问需要session的请求时,可以使用HttpServletRequest对象获取session,如下所示:
```
@RequestMapping("/user")
public String user(HttpServletRequest request) {
HttpSession session = request.getSession();
String username = (String) session.getAttribute("username");
String email = (String) session.getAttribute("email");
//...
return "index";
}
```
以上就是SpringBoot Redis session共享的实现步骤,可以有效解决负载均衡下session不一致的问题,并且提高了系统的性能和可靠性。
### 回答3:
Spring Boot 是一种快速开发Spring应用程序的框架和工具,可以大幅度提高开发效率。而 Redis 是一款高性能的内存数据存储系统,在分布式系统中被广泛应用。在Spring Boot应用程序中,Session区域可以实现Redis缓存的共享。这种共享方式可以允许多个应用程序同时访问相同的Session数据,从而提高应用程序的性能和可伸缩性。
使用Spring Boot Redis Session共享的步骤如下:
第一步:在导入Spring Boot应用程序中添加Redis的依赖,可以使用Maven或者Gradle来管理。
第二步:使用Spring Boot提供的RedisTemplate或者LettuceConnectionFactory来创建Redis连接。
第三步:使用Spring Boot提供的Session配置来开启Session Redis共享功能,在application.yml或者application.properties文件中添加如下配置:
`spring.session.store-type=redis`
`spring.redis.host=127.0.0.1`
`spring.redis.port=6379`
`spring.redis.password=password`
第四步:在应用程序中使用Session对象来存储和访问共享数据。
```
import org.springframework.session.Session;
import org.springframework.session.data.redis.RedisIndexedSessionRepository;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class RedisSessionController {
private final RedisIndexedSessionRepository sessionRepository;
public RedisSessionController(RedisIndexedSessionRepository sessionRepository) {
this.sessionRepository = sessionRepository;
}
@RequestMapping("/redis/session")
public String setSession() {
Session session = sessionRepository.createSession();
session.setAttribute("key", "value");
sessionRepository.save(session);
return "session";
}
@RequestMapping("/redis/session/read")
public String readSession() {
Session session = sessionRepository.findById("session_id");
Object value = session.getAttribute("key");
return value.toString();
}
}
```
在上述示例代码中,我们可以通过RedisIndexedSessionRepository创建Session对象,在设置Session值后通过save()方法将其存储到Redis缓存中。通过findById()方法可以检索Session对象,然后通过getAttribute()方法获取存储在Session中的值。
总结起来,Spring Boot Redis Session共享是非常方便和实用的方式。通过使用Redis缓存,应用程序可以获得更好的性能和可伸缩性,同时实现Session共享处理,使得开发人员可以更加轻松地实现分布式应用程序。
阅读全文
相关推荐
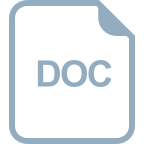
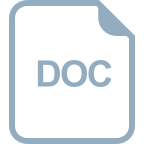
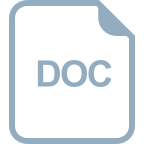
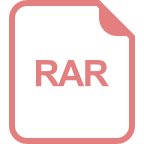
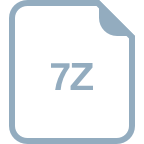
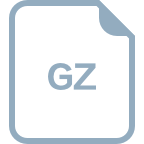
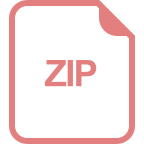
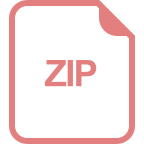
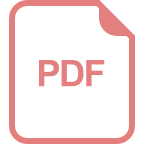
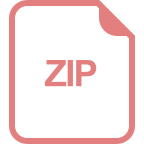
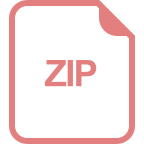
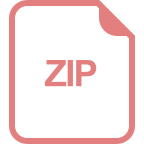
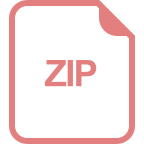
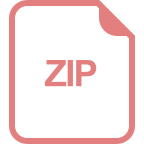
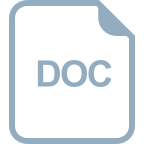



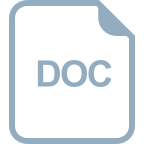