在C++中用vector存储TXT中不同类型数据
时间: 2024-02-22 11:58:29 浏览: 22
可以使用C++标准库中的`vector`容器来存储TXT中不同类型的数据。在读取TXT文件时,可以使用`ifstream`类打开文件,并使用`getline`函数逐行读取文件内容。在每一行中,可以使用字符串流`stringstream`来将字符串转换为不同类型的数据,然后将这些数据存储在`vector`容器中。
以下是一个示例代码,可以读取一个包含不同类型数据的TXT文件,并将其存储在`vector`容器中:
```c++
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
#include <string>
using namespace std;
int main() {
ifstream file("data.txt");
vector<int> ints;
vector<double> doubles;
vector<string> strings;
string line;
while (getline(file, line)) {
stringstream ss(line);
string word;
while (ss >> word) {
if (isdigit(word[0])) { // 如果第一个字符是数字,则将其转换为int或double类型
if (word.find('.') != string::npos) { // 如果包含小数点,则转换为double类型
doubles.push_back(stod(word));
} else {
ints.push_back(stoi(word));
}
} else { // 否则将其作为字符串类型存储
strings.push_back(word);
}
}
}
// 输出存储的数据
cout << "Ints:" << endl;
for (int i : ints) {
cout << i << " ";
}
cout << endl;
cout << "Doubles:" << endl;
for (double d : doubles) {
cout << d << " ";
}
cout << endl;
cout << "Strings:" << endl;
for (string s : strings) {
cout << s << " ";
}
cout << endl;
return 0;
}
```
在这个示例代码中,我们首先打开了一个名为"data.txt"的文件,并定义了三个类型为`vector`的容器,分别用于存储整数、双精度浮点数和字符串。然后,我们使用`getline`函数逐行读取文件内容,并使用字符串流`stringstream`将每一行分解为单词。对于每个单词,我们检查其第一个字符是否为数字,如果是,则将其转换为`int`或`double`类型,并存储在相应的容器中;否则,将其作为字符串类型存储。最后,我们输出存储的数据。
相关推荐
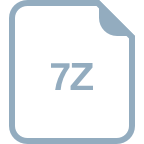
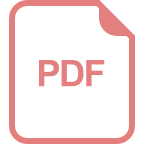














