基于AT89S52单片机实现PL2302串口转USB串口接口模块的软件代码,在Keil 4中
时间: 2023-06-14 18:07:39 浏览: 122
由于AT89S52没有USB接口,因此需要使用PL2302芯片将串口信号转换成USB信号。下面是一个基于AT89S52单片机实现PL2302串口转USB串口接口模块的软件代码。
首先,需要引入头文件及定义一些常量:
```c
#include <REGX52.H>
#define FOSC 11059200L
#define BAUD 9600
sbit TXD = P3^1;
sbit RXD = P3^0;
```
然后,需要定义一些变量:
```c
unsigned char uart_buf[16];
unsigned char uart_head = 0;
unsigned char uart_tail = 0;
```
接下来是初始化串口的函数:
```c
void init_uart()
{
TMOD &= 0x0F;
TMOD |= 0x20;
TH1 = TL1 = -(FOSC/12/32/BAUD);
TR1 = 1;
ES = 1;
EA = 1;
}
```
初始化PL2302芯片的函数:
```c
void init_pl2302()
{
P1 = 0x00;
P0 = 0xFF;
P1 = 0x80;
P0 = 0xBF;
}
```
发送一个字节的函数:
```c
void send_byte(unsigned char byte)
{
SBUF = byte;
while(!TI);
TI = 0;
}
```
发送一个字符串的函数:
```c
void send_string(unsigned char *str)
{
while(*str != '\0')
{
send_byte(*str++);
}
}
```
接收一个字节的函数:
```c
unsigned char recv_byte()
{
unsigned char byte;
while(!RI);
byte = SBUF;
RI = 0;
return byte;
}
```
主函数中的串口接收中断:
```c
void uart() interrupt 4 using 1
{
uart_buf[uart_head] = recv_byte();
uart_head = (uart_head + 1) % 16;
}
```
主函数中的循环:
```c
void main()
{
init_uart();
init_pl2302();
send_string("Hello World!\r\n");
while(1)
{
if(uart_head != uart_tail)
{
send_byte(uart_buf[uart_tail]);
uart_tail = (uart_tail + 1) % 16;
}
}
}
```
以上是基于AT89S52单片机实现PL2302串口转USB串口接口模块的软件代码。需要注意的是,在Keil 4中编译时需要选择8051系列的单片机。
阅读全文
相关推荐



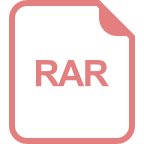
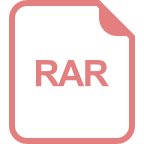


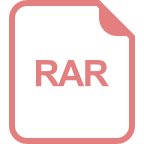







